With the release of .NET 8, Microsoft has introduced several new features and improvements to enhance performance and developer productivity. Among these, one of the most notable additions is the concept of "Frozen Collections." This article explores what frozen collections are, their benefits, and how to use them effectively in your .NET applications.
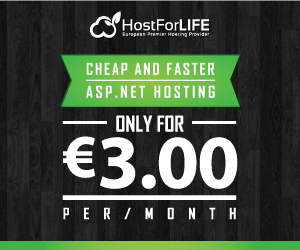
Frozen Collections: What Are They?
A new kind of immutable collection called frozen collections is intended to improve access speed and reduce memory usage in order to maximize performance. Frozen collections are intentionally made to be immutable from the beginning, in contrast to conventional read-only collections, which are frequently nested inside changeable collections. Once a collection is frozen, its immutability ensures that it cannot be altered, which has various performance benefits.
Key Benefits of Frozen Collections
1. Performance Optimization
The primary advantage of frozen collections lies in their performance. By guaranteeing immutability, the .NET runtime can make several optimizations.
- Memory Efficiency: Since frozen collections do not change, they can be stored in a compact form, reducing the overall memory footprint.
- Access Speed: Lookup operations are faster because the underlying data structure can be optimized for read access.
- Thread Safety: Frozen collections are inherently thread-safe, eliminating the need for synchronization mechanisms when accessing them concurrently.
2. Predictable Behavior
Immutable collections provide predictable behavior, which simplifies debugging and reasoning about code. When a collection is guaranteed not to change, developers can avoid common pitfalls related to concurrent modifications and side effects.
3. Simplified Code
Using frozen collections can lead to cleaner and more maintainable code. Since the collections cannot be altered, functions that operate on them do not need to account for modifications, leading to simpler and more readable implementations.
Using Frozen Collections in .NET 8
To use frozen collections in .NET 8, you can leverage the new FrozenCollection<T> class available in the System.Collections.Immutable namespace. Here’s a basic example to illustrate how to create and use a frozen collection.
using System;
using System.Collections.Immutable;
class Program
{
static void Main()
{
var mutableList = new List<int> { 1, 2, 3, 4, 5 };
// Create a frozen collection from the mutable list
var frozenCollection = mutableList.ToFrozenCollection();
// Attempting to modify the frozen collection will result in a compile-time error
// frozenCollection.Add(6); // Not allowed
// Accessing elements is fast and efficient
Console.WriteLine(frozenCollection[0]); // Output: 1
// The collection is inherently thread-safe
Parallel.ForEach(frozenCollection, item => {
Console.WriteLine(item);
});
}
}
public static class Extensions
{
public static FrozenCollection<T> ToFrozenCollection<T>(this IEnumerable<T> source)
{
var builder = new FrozenCollectionBuilder<T>();
foreach (var item in source)
{
builder.Add(item);
}
return builder.ToFrozenCollection();
}
}
Creating a Frozen Collection
In the example above, we start with a mutable list of integers. Using an extension method, we convert this list into a FrozenCollection<T>. The FrozenCollectionBuilder<T> class is used to add elements to the collection before it is frozen.
Accessing Elements
Once the collection is frozen, we can access its elements just like a regular collection. However, any attempt to modify the collection will result in a compile-time error, ensuring immutability.
Thread Safety
The inherent thread safety of frozen collections allows for safe concurrent access without additional synchronization, making them ideal for use in multi-threaded environments.
Conclusion
Frozen collections in .NET 8 represent a significant advancement in collection management, providing developers with powerful tools to create high-performance, immutable data structures. By leveraging these collections, you can achieve better memory efficiency, faster access times, and simplified code. As .NET continues to evolve, features like frozen collections will play a crucial role in enabling developers to build robust and efficient applications.