Response caching is the technique by which a browser or other client stores a server's response in memory. As a result, requests for the same resources will be processed more quickly in the future. Additionally, this will spare the server from processing and producing the same response over and over again.
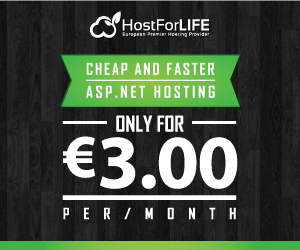
ASP.NET Core uses the ResponseCache property to set the response caching headers. Moreover, we can use the Response Caching Middleware to control the caching behavior from the server side. Once we've configured clients and other proxies to determine how to cache the server response, they can read the response caching headers. The HTTP 1.1 Response Cache Specification stipulates that browsers, clients, and proxies need to have caching headers.
Profiles in Cache
Instead of repeating the response cache settings on various controller action methods, we can create cache profiles and use them across the application. After it is configured, the application can utilize the cache profile's values as defaults for the ResponseCache attribute. Obviously, we can override the defaults by declaring the characteristics on the attribute.
A cache profile can be defined in the Program class.
builder.Services.AddControllers(options =>
{
options.CacheProfiles.Add("Default", new CacheProfile
{
Duration = 60,
Location = ResponseCacheLocation.Any
});
});
Here, we define a new cache profile named Default, whose location is set to public and whose duration is set to two minutes.
This cache profile can now be applied to any controller or endpoint.
[HttpGet("default")]
[ResponseCache(CacheProfileName = "Default")]
public IActionResult Default()
{
return Ok($"Default response was generated, {DateTime.Now}");
}
This will cause the response's defined cache-control settings to be applied.
cache-control: public,max-age=60
We can define multiple cache profiles in the app settings file and make the response cache settings configurable, avoiding hard-coding the cache settings in the Program class.
"CacheProfiles": {
"Cache5Mins": {
"Duration": 600,
"Location": "Any"
},
"CacheVaryByHeader": {
"Duration": 60,
"Location": "Any",
"VaryByHeader": "User-Agent"
}
}
The ConfigurationManager class can then be used to read the cache profiles in the Program class.
builder.Services.AddControllers(options =>
{
var cacheProfiles = builder.Configuration
.GetSection("CacheProfiles")
.GetChildren();
foreach (var cacheProfile in cacheProfiles)
{
options.CacheProfiles
.Add(cacheProfile.Key, cacheProfile.Get<CacheProfile>());
}
});
This approach to defining cache profiles is far superior, particularly if our application requires the definition of multiple cache profiles. Let’s run the application and validate the response cache.


The above screenshot is for the default response cache profile; the default value is 60 (1 min) until that response is the same; after that duration, the response is changed.

The cache vary by header response cache profile shown in the above example has a default value of 60 (1 minute), and the vary by header property value is User-Agent up until the point at which the answer changes.
Remember that while Response Caching can significantly improve your application's speed, it may not be suitable in all circumstances. Caching can cause unwanted or inappropriate behavior when user-specific data is served that shouldn't be cached, or when information is changing quickly.
Together, we developed and mastered the new method.
Have fun with coding!