
November 21, 2014 05:08 by
Peter
A Gridview is really a control for displaying and manipulating data from completely different data sources. It shows data from a sort of data sources inside a tabular format. Rather than boundfiled, I like to make use of TemplateField coz of the simplicity. In this article I am going to implement how to Add, Update, Delete selected record from GirdView control in ASP.NET 4.5.2
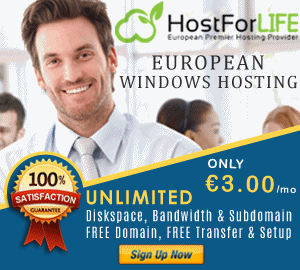
This the code for Add new record from footer and Update the selected row.
Default.aspx:
<asp:gridview allowpaging="True" autogeneratecolumns="False" cellpadding="4" forecolor="#333333" gridlines="None" id="gvstatus" onpageindexchanging="gvstatus_PageIndexChanging" onrowcancelingedit="gvstatus_RowCancelingEdit" onrowcommand="gvstatus_RowCommand" onrowediting="gvstatus_RowEditing" onrowupdating="gvstatus_RowUpdating" onselectedindexchanged="gvstatus_SelectedIndexChanged" runat="server" showfooter="True" width="600px">
<columns>
<asp:templatefield headerstyle-horizontalalign="Left" headertext="SrNo ">
<itemtemplate>
<%# Container.DataItemIndex + 1 %>
</itemtemplate>
</asp:templatefield>
<asp:templatefield headertext="ID" visible="false">
<itemtemplate>
<asp:label columnname_id="" id="lblid" runat="server" text="<%# Bind(">"> </asp:label>
</itemtemplate>
</asp:templatefield>
<asp:templatefield headertext="EmpName">
<itemtemplate>
<asp:label columnname_empname="" id="lblEmpName" runat="server" text="<%# Bind(">"></asp:label>
</itemtemplate>
<edititemtemplate>
<asp:textbox id="txtEmpName" runat="server" text="<%# Bind("columnname_EmpName") %>"></asp:textbox>
</edititemtemplate>
<footertemplate>
<asp:textbox id="txtfEmpName" runat="server"></asp:textbox>
</footertemplate>
</asp:templatefield>
<asp:templatefield headertext="empSalary">
<itemtemplate>
<asp:label id="lblempSalary" runat="server" text="<%# Bind("columnname_EmpSalary") %>"></asp:label>
</itemtemplate>
<edititemtemplate>
<asp:textbox id="txtempSalary" runat="server" text="<%# Bind("columnname_EmpSalary") %>"></asp:textbox>
</edititemtemplate>
<footertemplate>
<asp:textbox id="txtfempSalary" runat="server"></asp:textbox>
</footertemplate>
</asp:templatefield>
<asp:templatefield itemstyle-width="190px" showheader="False">
<itemtemplate>
<asp:button causesvalidation="False" commandname="Edit" id="btnedit" runat="server" text="Edit"></asp:button>
</itemtemplate>
<edititemtemplate>
<asp:button causesvalidation="True" commandname="Update" id="btnupdate" runat="server" text="Update"></asp:button>
<asp:button causesvalidation="False" commandname="Cancel" id="btncancel" runat="server" text="Cancel"></asp:button>
</edititemtemplate>
<footertemplate>
<asp:button commandname="Add" id="btnadd" runat="server" text="Add">
</asp:button></footertemplate>
</asp:templatefield>
</columns>
<pagerstyle backcolor="#A86E07" forecolor="White" horizontalalign="Center">
<selectedrowstyle backcolor="#E2DED6" font-bold="True" forecolor="#333333">
<headerstyle backcolor="#A86E07" font-bold="True" forecolor="White">
<editrowstyle backcolor="#d9d9d9">
<alternatingrowstyle backcolor="White" forecolor="#A86E07">
</alternatingrowstyle></editrowstyle></headerstyle></selectedrowstyle>
</pagerstyle>
</asp:gridview>
CodeBehind:
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
gvBind(); //Bind gridview
}
}
public void gvBind()
{
SqlDataAdapter dap = new SqlDataAdapter("select id, empName,empSalary from myTable", conn); DataSet ds = new DataSet();
dap.Fill(ds);
gvstatus.DataSource = ds.Tables[0];
gvstatus.DataBind();
}
Update the select row from girdview
protected void gvstatus_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
lblmsg.Text = "";
try
{
GridViewRow row = (GridViewRow)gvstatus.Rows[e.RowIndex];
Label lblid = (Label)gvstatus.Rows[e.RowIndex].FindControl("lblid");
TextBox txtname = (TextBox)gvstatus.Rows[e.RowIndex].FindControl("txtEmpName");
TextBox txtSalary = (TextBox)gvstatus.Rows[e.RowIndex].FindControl("txtempSalary");
string empName = txtname.Text;
string empSalary = txtSalary.Text;
string lblID=lblid.Text;
int result = UpdateQuery(empName, empSalary,lblID);
if (result > 0)
{
lblmsg.Text = "Record is updated successfully.";
}
gvstatus.EditIndex = -1;
gvBind();
}
catch (Exception ae)
{
Response.Write(ae.Message);
}
}
And this is the Code that I used to add new record into database form GirdView footer
protected void gvstatus_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName == "Add")
{
string empName = ((TextBox)gvstatus.FooterRow.FindControl("txtfempName")).Text;
string empSalry = ((TextBox)gvstatus.FooterRow.FindControl("txtfempSalary")).Text;
int result = InsertNewRecord(empName, empSalry);
if (result > 0)
{
lblmsg.Text = "Record is added successfully.";
}
gvstatus.EditIndex = -1;
gvBind();
}
}
protected void gvstatus_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
gvstatus.EditIndex = -1;
gvBind();
}
protected void gvstatus_RowEditing(object sender, GridViewEditEventArgs e)
{
lblmsg.Text = "";
gvstatus.EditIndex = e.NewEditIndex;
gvBind();
}
public void UpdateQuery(string empName, string empSalary, string lblID)
{
SqlCommand cmd = new SqlCommand("update myTable set empName='" + empName + "',empSalary='" + empSalary + "' where id='" + lblID + "'", conn);
conn.Open();
int temp = cmd.ExecuteNonQuery();
conn.Close();
return temp;
}
public void InsertNewRecord(string empName, string empSalary)
{
SqlCommand cmd = new SqlCommand("your insert query ", conn);
conn.Open();
int temp = cmd.ExecuteNonQuery();
conn.Close();
return temp;
}