When bulding professional web applications, there can be so many emails to be sent to users and writing the templates for each of this email inside code is not a good move. Here we can use email templates. First create a folder some where in your application directory as "MailTemplates" or some other suitable name. Now create a text file or html file for template.
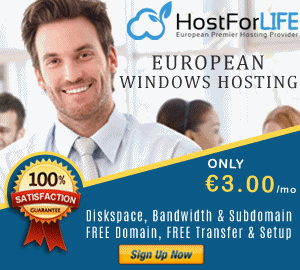
In this example, we are creating a sample template named welcome.htm:
Hi, <%=name%>
This is a sample mail template for demonstrating usage of email templates in asp.net
<a href="<%=url%>">Click here to read the article</a>
<a href="<%=rooturl%>">http://example.com/</a>
Now, we are creating an email utility for preparing and sending emails:
public static void SendMail(string fromAddress, string toAddress, string subject, string body)
{
using (MailMessage mail = BuildMessageWith(fromAddress, toAddress.Replace(',', ';'), subject, body))
{
SendMail(mail);
}
}
public static void SendMail(MailMessage mail)
{
try
{
SmtpClient smtp = new SmtpClient("your smtp host",port);
smtp.Send(mail);
}
catch (Exception e)
{
}
}
//build a mail message
private static MailMessage BuildMessageWith(string fromAddress, string toAddress, string subject, string body)
{
MailMessage message = new MailMessage
{
Sender = new MailAddress(Settings.WebMasterEmail), // on Behave of When From differs
From = new MailAddress(fromAddress),
Subject = subject,
Body = body,
IsBodyHtml = true,
};
string[] tos = toAddress.Split(';');
foreach (string to in tos)
{
message.To.Add(new MailAddress(to));
}
return message;
}
// read the text in template file and return it as a string
private static string ReadFileFrom(string templateName)
{
string filePath = System.Web.HttpContext.Current.Server.MapPath("~/MailTemplates/"+templateName);
string body = File.ReadAllText(filePath);
return body;
}
// get the template body, cache it and return the text
private static string GetMailBodyOfTemplate(string templateName)
{
string cacheKey = string.Concat("mailTemplate:", templateName);
string body;
body = (string)System.Web.HttpContext.Current.Cache[cacheKey];
if (string.IsNullOrEmpty(body))
{
//read template file text
body = ReadFileFrom(templateName);
if (!string.IsNullOrEmpty(body))
{
System.Web.HttpContext.Current.Cache.Insert(cacheKey,body, null,DateTime.Now.AddHours(1),System.Web.Caching.Cache.NoSlidingExpiration);
}
}
return body;
}
// replace the tokens in template body with corresponding values
private static string PrepareMailBodyWith(string templateName, params string[] pairs)
{
string body = GetMailBodyOfTemplate(templateName);
for (var i = 0; i < pairs.Length; i += 2)
{
body = body.Replace("<%={0}%>".FormatWith(pairs[i]), pairs[i + 1]);
}
return body;
}
public static string FormatWith(this string target, params object[] args)
{
return string.Format(Constants.CurrentCulture, target, args);
}
Now let's send the email:
string subject = "Welcome";
string body = PrepareMailBodyWith("welcome.htm", "name", "Anoop", "url", "http://waytocoding.blogspot.com/","rooturl","http://waytocoding.blogspot.com/");
SendMail("[email protected]", "[email protected]", subject, body);
HostForLIFE.eu ASP.NET 4.6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
