
November 9, 2016 08:55 by
Peter
In this post, you will learn how to upload a file without PostBack in ASP.NET. My main focus to write this blog is that, if you work with file upload control, the page requires full PostBack and if your file upload control is inside the update panel, you must specify your submit button in trigger, as shown below.
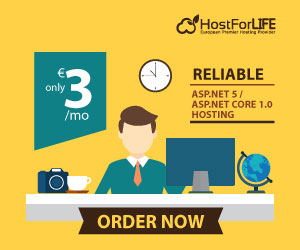
<Triggers>
<asp:PostBackTrigger ControlID=""/>
</Triggers>
This also causes the full PostBack problem. To remove this problem, use "Handler" in ASP. NET C#.
UploadFile.ashx Code
using System;
using System.Web;
using System.IO;
using System.Web.SessionState;
public class UploadFile: IHttpHandler, IRequiresSessionState {
public void ProcessRequest(HttpContext context) {
string filedata = string.Empty;
if (context.Request.Files.Count > 0) {
HttpFileCollection files = context.Request.Files;
for (int i = 0; i < files.Count; i++) {
HttpPostedFile file = files[i];
if (Path.GetExtension(file.FileName).ToLower() != ".jpg" &&
Path.GetExtension(file.FileName).ToLower() != ".png" &&
Path.GetExtension(file.FileName).ToLower() != ".gif" &&
Path.GetExtension(file.FileName).ToLower() != ".jpeg" &&
Path.GetExtension(file.FileName).ToLower() != ".pdf"
) {
context.Response.ContentType = "text/plain";
context.Response.Write("Only jpg, png , gif, .jpeg, .pdf are allowed.!");
return;
}
decimal size = Math.Round(((decimal) file.ContentLength / (decimal) 1024), 2);
if (size > 2048) {
context.Response.ContentType = "text/plain";
context.Response.Write("File size should not exceed 2 MB.!");
return;
}
string fname;
if (HttpContext.Current.Request.Browser.Browser.ToUpper() == "IE" || HttpContext.Current.Request.Browser.Browser.ToUpper() == "INTERNETEXPLORER") {
string[] testfiles = file.FileName.Split(new char[] {
'\\'
});
fname = testfiles[testfiles.Length - 1];
} else {
fname = file.FileName;
}
//here UploadFile is define my folder name , where files will be store.
string uploaddir = System.Configuration.ConfigurationManager.AppSettings["UploadFile"];
filedata = Guid.NewGuid() + fname;
fname = Path.Combine(context.Server.MapPath("~/" + uploaddir + "/"), filedata);
file.SaveAs(fname);
}
}
context.Response.ContentType = "text/plain";
context.Response.Write("File Uploaded Successfully:" + filedata + "!");
//if you want to use file path in aspx.cs page , then assign it in to session
context.Session["PathImage"] = filedata;
}
public bool IsReusable {
get {
return false;
}
}
}
web.config code
<?xml version="1.0"?>
<!--
For more information on how to configure your ASP.NET application, please visit
http://go.microsoft.com/fwlink/?LinkId=169433
-->
<configuration>
<system.web>
<compilation debug="true" targetFramework="4.0" />
</system.web>
<appSettings>
<add key="Upload" value="UploadFile" />
</appSettings>
</configuration>
Default.aspx code
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN""http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<%-- Should have internet connection for loading this file other wise inherit own js file for supporting js library--%>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script type="text/javascript">
function onupload() {
$(function() {
var fileUpload = $('#<%=FileUpload.ClientID%>').get(0);
var files = fileUpload.files;
var test = new FormData();
for (var i = 0; i < files.length; i++) {
test.append(files[i].name, files[i]);
}
$.ajax({
url: "UploadFile.ashx",
type: "POST",
contentType: false,
processData: false,
data: test,
success: function(result) {
if (result.split(':')[0] = "File Uploaded Successfully") {
document.getElementById("<%=lbl_smsg.ClientID%>").innerText = result.split(':')[0];
} else {
document.getElementById("<%=lbl_emsg.ClientID%>").innerText = result;
}
},
error: function(err) {
alert(err.statusText);
}
});
})
}
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:ScriptManager ID="scmain" runat="server"></asp:ScriptManager>
<asp:UpdatePanel ID="upmain" runat="server">
<ContentTemplate>
<fieldset>
<legend>Upload File WithOut PostBack inside Update Panel</legend>
<asp:FileUpload ID="FileUpload" runat="server" />
<input type="button" id="btnUpload" value="Upload Files" onclick="onupload();" />
<asp:Label ID="lbl_emsg" runat="server" ForeColor="Red"></asp:Label>
<asp:Label ID="lbl_smsg" runat="server" ForeColor="Green"></asp:Label>
</fieldset>
</ContentTemplate>
</asp:UpdatePanel>
</form>
</body>
</html>
Default.aspx code.cs code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default: System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e) {}
}
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
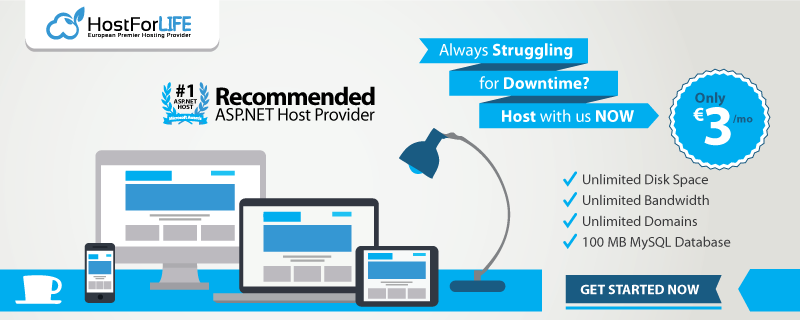