
August 15, 2023 10:34 by
Peter
Asynchronous operations and lazy data streaming are two fundamental ideas in C# programming that help developers design efficient and responsive apps. While there is no straight "async yield" term in C#, you can combine the power of async and yield to achieve equivalent behavior. In this post, we will look at how to efficiently stream data by using asynchronous generators with async functions and iterators.
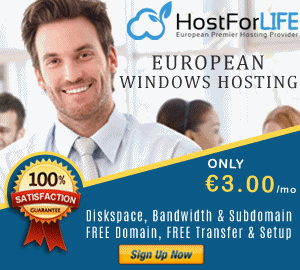
Lazy Loading and Asynchronous Programming
Before we get started, let's go through the two main principles we'll be working with:
Programming in an Asynchronous Environment
Asynchronous programming allows you to run tasks at the same time without interrupting the main thread. In C#, the async and await keywords make it easier to design code that waits for asynchronous activities to complete, which improves application responsiveness.
Loading Time and Yield
Lazy loading is a strategy that loads data only when it is required. In C#, the yield keyword is used to generate iterators, which allow for lazy data loading in a memory-efficient manner. It produces elements on the fly, which saves memory and improves efficiency.
Asynchronous Generator Design
We will develop asynchronous generators using a combination of async methods and iterators to combine asynchronous programming and lazy loading. This is how it works:
Asynchronous Iterator Method Definition
To begin, we will write an asynchronous procedure that uses the yield return statement to generate items. This method will include asynchronous actions as well as the yield keyword.
internal class AsyncGeneretors
{
public static async IAsyncEnumerable<int> GenerateNumbersAsync()
{
for (int i = 0; i < 10; i++)
{
await Task.Delay(100); // Simulate asynchronous work
yield return i;
}
}
}
Note. IAsyncEnumerable was introduced in C# 8.0. It is a feature that allows you to work with asynchronous sequences of data in a more convenient and efficient manner. It is used in scenarios where you want to represent and process collections of data that are produced asynchronously, such as when working with streams, databases, or other asynchronous data sources.
Consuming the Asynchronous Generator:
To consume the asynchronous generator, we'll use the await foreach statement. This allows us to asynchronously iterate over the generated elements without blocking the main thread.
using AsyncYield;
Console.WriteLine("Combine Async and Yield");
var numbers = AsyncGeneretors.GenerateNumbersAsync();
await foreach (var number in numbers )
{
Console.WriteLine(number);
}
Console.Read();
Benefits and Use Cases
Combining async and yield provides several benefits for data streaming and processing.
- Memory Efficiency: Asynchronous generators load and process data lazily, reducing memory consumption. This is especially useful when dealing with large datasets.
- Responsive Applications: By leveraging asynchronous programming, your application remains responsive even when performing time-consuming tasks.
- Parallelism: Asynchronous operations can execute concurrently, allowing for efficient utilization of available resources.
- Real-time Data: Asynchronous generators are well-suited for scenarios where data is constantly changing or being updated in real time.
Conclusion
While C# does not offer a built-in "async yield" keyword, you can achieve similar behavior by combining async methods and the yield keyword. This approach enables you to create asynchronous generators that efficiently stream data while keeping your application responsive. By understanding and leveraging the power of asynchronous programming and lazy loading, you can build high-performance, memory-efficient applications that handle data streaming seamlessly. Happy coding!