Once a string type is generated in C#, its contents cannot be changed since the string type is immutable. This suggests that modifying a string-type object after it has been created will cause the object to be constructed again in memory as a new instance. Additionally, changing the string frequently could cause performance problems.
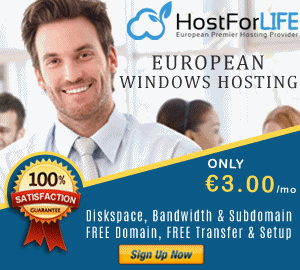
StringBuilder solves this problem because, unlike string, it can dynamically increase its memory to support any kind of manipulation.
How to make a StringBuilder correctly?
It is not too difficult to instantiate a StringBuilder. We can instantiate a class by using the new keyword to create an instance of that class. A StringBuilder also contains other constructors.

Conversion of StringBuilder to String
We can use a StringBuilder for any type of string manipulation. But the StringBuilder does not return a string. Therefore, in order to retrieve a string, we must use the ToString() method.
var sb = new StringBuilder("Peter Scott");
var name = sb.ToString();
The content is first converted to a string and then a StringBuilder is created with a default text.
StringBuilder Techniques
We can work with a StringBuilder's contents in a few different ways. These include Replace(), Insert(), Clean(), Remove(), Append(), AppendLine(), and AppendFormat().
Append
A new string is appended to the end of the existing StringBuilder using the Append() function. The StringBuilder's length can be doubled, and space allocation happens automatically.
var sb = new StringBuilder("Hello, ");
sb.Append("Peter Scott");
var name = sb.ToString();
Console.WriteLine("{0} - {1}", nameof(name), name);
AppendLine
AppendLine() is useful when we want to add a line terminator to our StringBuilder. To accomplish this, we can make a StringBuilder and use the following method.
var sb = new StringBuilder("Hello, ");
sb.Append("Peter Scott");
sb.AppendLine();
sb.AppendLine("How are you?");
Console.WriteLine(sb.ToString());
AppendFormat
AppendFormat() appends a string to the end of StringBuilder in a predetermined format. The conventions of either the chosen culture or the current system culture are revealed in the produced string. The method lets us pass the desired format for our string as an input.
var sb = new StringBuilder("Hello, ");
sb.Append("Peter Scott");
var text = "C# Corner Rank";
var rank = 120;
sb.AppendLine();
sb.AppendFormat("{0} - {1}", text, rank);
Console.WriteLine(sb.ToString());
This method adds, to our StringBuilder object, a string, substring, character array, part of a character array, or the string representation of a primitive data type at a certain place. We may also add the index for our insertion using this method.
var sb = new StringBuilder("Hello");
var name = ", Peter Scott";
sb.Insert(5, name);
Console.WriteLine(sb.ToString());
Replace
Several character occurrences in the StringBuilder object are replaced by this method. The desired character sequence and a new value are entered into the method as inputs.
var sb = new StringBuilder("Hello");
var name = ", Peter Scott";
sb.Insert(5, name);
Console.WriteLine("Orioginal data: {0}", sb.ToString());
Console.WriteLine();
sb.Replace("Lee", "Cooper");
Console.WriteLine("Replace data: {0}", sb.ToString());
Replace
A predetermined amount of characters are removed from the StringBuilder using the Remove() function. The start index and the number of characters to be deleted are the input parameters.
var sb = new StringBuilder("Hello");
sb.Append(", Peter Scott");
Console.WriteLine("Orioginal data: {0}", sb.ToString());
Console.WriteLine();
sb.Remove(0, 7);
Console.WriteLine("Remove data: {0}", sb.ToString());
Remove
The Remove technique eliminates 7 characters starting at index 0.
Clear
The StringBuilder object's characters are all eliminated using this function. This method reduces the length of a StringBuilder to zero.
var sb = new StringBuilder("Hello");
sb.Append(", Peter Scott");
Console.WriteLine("Orioginal data: {0}", sb.ToString());
Console.WriteLine();
sb.Clear();
Console.WriteLine("Clear data: {0}", sb.ToString());
Clear
We learned the new technique and evolved together.
Happy coding!