In this post, I tell you how to invoke an ASP.NET page method directly from your own AJAX library. A Page method is a method that is written directly in a page. It is generally called when the actual page is posted back and some event is raised from the client. The pageMethod is called directly from ASP.NET engine. To implement PageMethod, first you need to annotate our method as WebMethod. A WebMethod is a special method attribute that exposes a method directly as XML service.
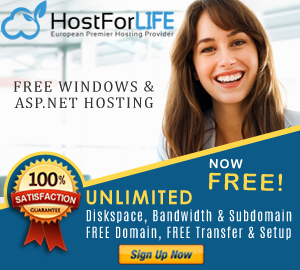
Here are the steps to create the application:
Step 1
Start a new ASP.NET Project.
Step 2
Add JQuery to your page. Then, you can add a special JQuery plugin which stringify a JSON object. And post the codes like below :
(function ($) {
$.extend({
toJson: function (obj) {
var t = typeof (obj);
if (t != "object" || obj === null) {
// simple data type
if (t == "string") obj = '"' + obj + '"';
return String(obj);
}
else {
// recurse array or object
var n, v, json = [], arr = (obj && obj.constructor == Array);
for (n in obj) {
v = obj[n]; t = typeof (v);
if (t == "string") v = '"' + v + '"';
else if (t == "object" && v !== null) v = JSON.stringify(v);
json.push((arr ? "" : '"' + n + '":') + String(v));
}
return (arr ? "[" : "{") + String(json) + (arr ? "]" : "}");
}
}
});
// extend plugin scope
$.fn.extend({
toJson: $.toJson.construct
});
})(jQuery);
The code actually extends JQuery to add a method called toJSON to its prototype.
Step 3
Add the server side method to the Default.aspx page. For simplicity, you can actually return the message that is received from the client side with some formatting.
[WebMethod]
public static string DisplayTime(string message)
{
// Do something
return string.Format("Hello ! Your message : {0} at {1}", message, DateTime.Now.ToShortTimeString());
}
You should make this method static, and probably should return only serializable object.
Step 4
Add the following Html which actually puts one TextBox which takes a message and a Button to call server.
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<h2>
Welcome to ASP.NET!
</h2>
<p>
Write Your message Here : <input type="text" id="txtMessage" />
</p>
<p>
<input type="button" onclick="javascript:callServer()" value="Call Server" />
</p>
</asp:Content>
Once you add this html to your default.aspx page, add some javascript to the page. We add the JQuery and our JSONStringify code to it.
<script src="Scripts/jquery-1.4.1.min.js" type="text/javascript"></script>
<script src="Scripts/JSONStringify.js" type="text/javascript"></script>
<script type="text/javascript">
function callServer() {
var objdata = {
"message" : $("#txtMessage").val()
};
$.ajax({
type: "POST",
url: "Default.aspx/DisplayTime",
data: $.toJson(objdata),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (msg) {
alert(msg.d);
},
error: function (xhr, ajaxOptions) {
alert(xhr.status);
}
});
}
</script>
The above code actually invokes a normal AJAX call to the page. You can use your own library of AJAX rather than JQuery to do the same.
Hope it works for you!
Free ASP.NET Hosting
Try our Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc. You will not be charged a cent for trying our service for the next 3 days. Once your trial period is complete, you decide whether you'd like to continue.
