Building scalable and reliable apps is now simpler than ever thanks to.NET 8 and Entity Framework Core (EF Core). Making models that map to database tables is a crucial step in developing a data-driven application because it enables object-oriented data manipulation. This post will demonstrate how to use.NET 8 and Entity Framework Core to create a model and link it to a database table.
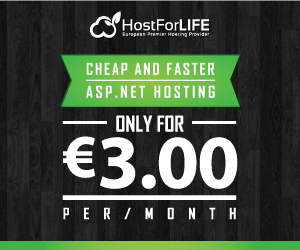
Prerequisites
Before we dive in, make sure you have the following set up.
- .NET SDK 8.0 or higher.
- Visual Studio or VS Code installed. In my scenario, I will develop the project in Visual Studio.
- Basic knowledge of C# and object-oriented programming.
Step 1. Setting Up the Project
First, create a new .NET 8 project. In this example, we'll make an ASP.NET Core Web API project, but the process is similar for other types of .NET applications.
Open Visual Studio and click on Create a new project. Select ASP.NET Core Web API, and click on the Next button.

Create a project as an E-POS and the Solution name as an E-Business. Click on the Next button.|

After clicking on the create button, the E-POS project was created.

Now, I'm going to create the Models folder in my project. Right-click on your project to open a pop-up menu, then click 'Add,' select 'New Folder,' and name it 'Models.'

Inside the Models folder, create a new class file. Right-click on the Models folder, select 'Add,' then choose 'Class,' click it, and give it the name ‘Product’.
Step 2. Define the Model Class
A model in Entity Framework Core is simply a C# class that defines the structure of a database table. Let's define a simple product model with properties for Id, Name, Price, and CreatedDate.
Create a new folder called Models, and inside it, add a file named Product.cs.
namespace E_POS.Models
{
public class Product
{
public int Id { get; set; } // Primary Key
public required string Name { get; set; }
[Column(TypeName = "decimal(18, 2)")]
public decimal Price { get; set; }
public DateTime CreatedDate { get; set; }
}
}

‘Id’ is the primary key, which EF Core will map to the table's primary key by convention.
Other properties (Name, Price, and CreatedDate) will be mapped to corresponding columns in the table.
Install Required NuGet Packages
To install NuGet packages like those required for Entity Framework Core. There are multiple ways, depending on your environment and tools. Here's a summary of the different methods you can use:
1. Using .NET CLI (Command Line Interface)
This is the method you mentioned, using the terminal or command prompt. It’s a simple and effective way to add packages to your project.
For Microsoft.EntityFrameworkCore
Install-Package Microsoft.EntityFrameworkCore
For Microsoft.EntityFrameworkCore.Tools
Install-Package Microsoft.EntityFrameworkCore.Tools
For Microsoft.EntityFrameworkCore.Design
Install-Package Microsoft.EntityFrameworkCore.Design
For Microsoft.EntityFrameworkCore.SqlServer (to work with SQL Server)
Install-Package Microsoft.EntityFrameworkCore.SqlServer

2. Using NuGet Package Manager in Visual Studio
Visual Studio offers a graphical interface to manage NuGet packages.
Steps
- Right-click on your project in Solution Explorer.
- Select Manage NuGet Packages.
- In the Browse tab, search for the required packages (e.g., Microsoft.EntityFrameworkCore, Microsoft.EntityFrameworkCore.Tools, etc.).
- Click Install for each package.
3. Using the NuGet Package Manager Console in Visual Studio
This console allows you to install packages using commands similar to the CLI but directly within Visual Studio.
Check whether the packages are installed or not.

Step 3. Configure the Connection String
Now, let's configure the database connection string.
Open the appsettings.json file and add your database connection details under the ConnectionStrings section.
{
"ConnectionStrings": {
"DefaultConnection": "Server=LAPTOP-A40000TJ\\SQLEXPRESS; Database=EBusiness; Integrated Security=True; Encrypt=True; TrustServerCertificate=True;"
}
}
This connection string defines how a .NET application connects to a SQL Server database. It specifies,
- Server: LAPTOP-A4E1RITJ\SQLEXPRESS (machine name and SQL instance)
- Database: E_Business
- Integrated Security: Uses Windows Authentication.
- Encrypt: Secures data transmission.
- TrustServerCertificate: Accepts self-signed SSL certificates.

This connection string assumes you are using SQL Server. Replace the placeholders with your actual database credentials.
Step 4. Create the DbContext Class
The DbContext class in Entity Framework Core manages the database connection and is responsible for querying and saving data. Let’s create the AppDbContext class that represents our database session and exposes the Product model as a DbSet.
In the root of your project, create a folder named Data and add a class file called AppDbContext.cs.
using E_POS.Models;
using Microsoft.EntityFrameworkCore;
namespace E_POS.Data
{
public class AppDbContext: DbContext
{
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options)
{
}
public DbSet<Product> Products { get; set; }
}
}

DbSet<Product> represents the Products table in the database.
The AppDbContext constructor accepts DbContextOptions to configure the database connection.
Step 5. Register the DbContext in the Program.cs
To ensure that Entity Framework Core can access your DbContext, register it in the Program.cs file. Modify the Program.cs to include AppDbContext.
#region Database Configure
var connectionString = builder.Configuration.GetConnectionString("DefaultConnection");
builder.Services.AddDbContext<AppDbContext>(options => options.UseSqlServer(connectionString));
#endregion

Step 6. Create and Apply Migrations
Migrations allow you to create or modify the database schema to match your models.
In Visual Studio, you can open the Package Manager Console by following these steps.
- Go to the Tools menu at the top of Visual Studio.
- Select NuGet Package Manager.
- Click on Package Manager Console.

To make an initial migration and apply it, run the following commands.
Add-Migration InitialCreate
Database-Update

Add-Migration InitialCreate: Creates a new migration based on the Product model and AppDbContext.
Database-Update: Applies the migration to your database, creating the necessary tables.
Let’s check whether the database is created or not.


Summary
In this article, we’ve explored how to create a model and map it to a database table in .NET 8 using Entity Framework Core. The process involves defining a model, creating a DbContext, configuring the database connection, and using migrations to keep your database schema in sync with your models.