
November 5, 2019 10:53 by
Peter
HTTP is a stateless protocol, so we need some mechanism to maintain our App State. Server Side Session has been a way to maintain our state on the server side. In this article we'll see what differences ASP.NET Core has introduced regarding SESSION.
We'll quickly discuss how we used to use Sessions before ASP.NET Core and then we'll see how to access Sessions in ASP.NET Core.
Session In Pre-ASP.NET Core era
You get Session functionality by default (without adding any package)
Previously, you would have accessed Session by -
Session variable in your Controllers/Forms
System.Web.HttpContext.Current.Session in places where you don't have direct access to the Session variable.
Anything you store in session is stored as Object. You store values in Key/Value format.
Session["mydata"] = 10;
Or to access on those places where Session is not available (e.g. Non-Controller classes)
System.Web.HttpContext.Current.Session["mydata"] = 10;
Quite difficult to mock Session Object for Unit Testing
Session in ASP.NET Core 2.2
Now, Session is not available by default.
You need to add the following package. Meta package by default provides you this.
<PackageReference Include="Microsoft.AspNetCore.Session" Version="2.2.0" />
In Startup.ConfigureServices, you need to add the following to register services with DI Container.
services.AddDistributedMemoryCache();//To Store session in Memory, This is default implementation of IDistributedCache
services.AddSession();
In Startup.Configure, you need to add the following (before UseMVC) to add Session Middleware.
app.UseCookiePolicy();
app.UseSession();
app.UseMvc(routes =>
Make sure the following is also there (It is added by default when you use ASP.NET Core MVC Template).
app.UseCookiePolicy();
ASP.NET Core 2.2 onwards adds Cookie Consent (true) in the Startup file. When an application runs, the user needs to accept Cookie Consent on screen. When the user accepts the policy on the page, it creates a consent cookie. It is to follow GDPR and to give control to the user if the user wants to store cookies from a site or not. If the user doesn't accept that, Session does not work because Session requires a cookie to send/receive session Id. You may face this issue while working with ASP.NET Core MVC default template.
How to access Session in Controller?
You will notice that you don't have "Session" variable available now. Controller now has a property "HttpContext" which has "Session" variable. So, you can access session in controller by using the following code.
var a = this.HttpContext.Session.GetString("login");
HttpContext.Session.SetString("login", dto.Login);
How to access Session in Non-Controller class?
Now, you don't have System.Web.HttpContext.Current.Session in ASP.NET Core. To access session in non-controller class -
First, register the following service in Startup.ConfigureServices;
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
Now, register a class (example - TestManager) where you want to access the Session in Startup.ConfigureServices;
services.AddScoped<TestManager>();
Note
You may use AddTransient or AddSingleton according to your logic.
Now, in TestManager class, add the following code.
private readonly IHttpContextAccessor _httpContextAccessor;
private readonly ISession _session;
public TestManager(IHttpContextAccessor httpContextAccessor)
{
_httpContextAccessor = httpContextAccessor;
_session = _httpContextAccessor.HttpContext.Session;
}
The above code is receiving IHttpContextAccessor object through dependency injection and then, it is storing Sessions in a local variable.
How to access Session in View file?
Add the following at the top of your View file.
@using Microsoft.AspNetCore.Http
@inject IHttpContextAccessor httpContextAccessor
Then, you may access the Session variable in your View like following.
@httpContextAccessor.HttpContext.Session.GetString("login")
What else is changed regarding Session?
Session is non-locking now.
A session is not created until you have at least one value in it
You need to use functions to get & set data. Array syntax is not supported now.
Now, ISession only provides Get & Set method which takes & returns data in Byte Array format.
If you want to store data in the String format, you may add the following in your file and use extension methods.
using Microsoft.AspNetCore.Http;
It exposes the new extension methods.
SetInt32
GetInt32
SetString
GetString
Under the hood, these covert the data into bytes.
You may also write your own extension methods. For example, the following Extension Methods help you store & retrieve any complex type.
public static class SessionExtensions
{
public static void Set<T>(this ISession session, string key, T value)
{
session.Set<(key, JsonConvert.SerializeObject(value));
}
public static T GetObject<T>(this ISession session, string key)
{
var value = session.GetString(key);
return value == null ? default(T) : JsonConvert.DeserializeObject<T>(value);
}
}
In the next article, we'll learn about SESSION Wrapper Design Pattern.
Summary
Session concepts are similar to what we've seen in earlier .NET Frameworks. The real difference is that now, it is cleaner & more flexible to use.
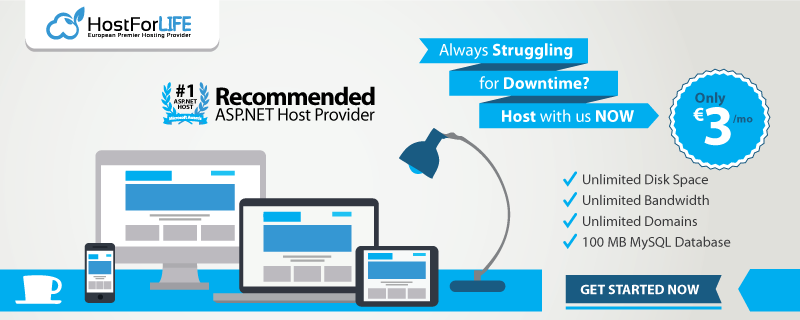