In this post, we will consume the service from a .NET client and a web page.
Consuming Web API OData using a .NET client:
A Web API OData service can be consumed using WCF Data Services client. If you gave already worked with WCF Data Services, you already know about consuming Web API OData Service as well.
Right click on a .NET project and choose the option Add Service Reference. In the dialog, enter the OData service URL and click the Go button.
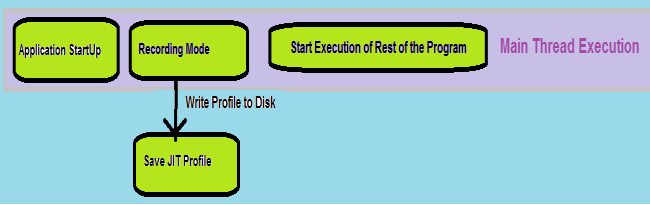
The dialog parses the metadata received from the server and shows the available entities under container as shown in the screenshot. As we created just one entity in the service, we see the entity Employee alone. Name the namespace as you wish and hit OK. Visual Studio generates some classes for the client application based on the metadata.
The generated code contains the following:
- A Container class, which is responsible for communicating with the service. It holds DataServiceQuery<TEntity> type properties for each EntitySet on the server
- A class for every entity type. This class contains all properties mapped on the server, information about key of the entity
A Container is much like a DbContext in Entity Framework. It handles all the operations. Container is responsible for building OData URLs and sending requests to the service for any operation that client asks for. Let’s start by creating a Container. Constructor of the container accepts a URI, which is base address of the Web API OData service.
Container container = new Container(new Uri("http://localhost:1908/odata"));
To fetch details of all employees, we need to invoke the Corresponding DataServiceQuery property.
var employees = container.Employees;
Although the statement looks like an in-memory operation, it generates the corresponding URL internally and calls the server. Similarly, to get details of an employee with a given Id, we can write a LINQ query as shown:
var employee = container.Employees.Where(e => e.Id == 3).FirstOrDefault();
The above query makes a call to the Get method accepting key in the Web API Controller.
To create a new employee, we need to create an object of the Employee class, add it and ask Container to save it. Following snippet demonstrates this:
Employee emp = new Employee() { Id = 0, Name = "Hari", Salary = 10000 };
container.AddToEmployees(emp);
container.SaveChanges();
Performing update is also much similar. The difference is with calling the SaveChanges method.
emp = container.Employees.Where(e => e.Id == 3).FirstOrDefault();
emp.Name = "Stacy";
container.UpdateObject(emp);
container.SaveChanges(SaveChangesOptions.ReplaceOnUpdate);
If SaveChanges is called with SaveChangesOptions.ReplaceOnUpdate, it performs PUT operation on the resource. If SaveChangesOptions.PatchOnUpdate is passed, it performs PATCH operation on the resource.
To delete an entry, we need to pass an object to DeleteObject method and just like earlier cases; we need to call the SaveChanges method on the Container.
container.DeleteObject(container.Employees.Where(e => e.Id == 3).FirstOrDefault());
container.SaveChanges();
Consuming Web API OData using JavaScript client:
To consume the Web API OData service from a web page, the service has to be called using AJAX. The client can send an AJAX request to the URL of the OData service by specifying an HTTP verb to operate on the resource. To make our life easier, let’s use jQuery for AJAX calls.
To get details of all employees, we need to send a GET request to the OData URL. Values of entries in the collection are stored in a property named value in the object received as response. Fetching details of an employee with a given Id also follows similar approach. Following snippet demonstrates this:
$.getJSON(“/odata/Employees”, function(data){
$.each(data.value, function(){
//Modify UI accordingly
});
});
$.getJSON(“/odata/Employees(3)”, function(data){
//Modify UI accordingly
});
To add a new employee, we need to send the new object to $.post along with the URL.
var employee = {
"Id": 0,
"Name": “Ravi”,
"Salary": 10000
};
$.post(“/odata/Employees”, employee).done(function(data){
//Modify UI accordingly
});
Unfortunately, jQuery doesn’t have a shorthand method for PUT. But it is quite easy with $.ajax as well. To perform PUT on the resource, the request should be sent to the specific address with an ID and the modified object should be passed with the request.
var employee = {
"Id": 3,
"Name": “Ravi”,
"Salary": 10000
};
$.ajax({
url: "/odata/Employees(" + employee.Id + ")",
type: "PUT",
data: employee
});
Building request for DELETE is similar to put, we just don’t need to pass the object.
$.ajax({
url: "/odata/Employees(" + id + ")",
type: "DELETE"
});