
June 22, 2023 07:55 by
Peter
What are filters and what are their advantages?
Filters allow us to execute a piece of code before or after any request processing pipeline stage.
The advantages of filtration
- Instead of writing code for each individual action, we write it once and then reuse it.
- Extensible: They allow us to perform some action ABC before the method and some operation XYZ after the method.
Varieties of Filters
- Authorization Filters: Most prominent in ascending order to determine if the user is authorized.
- Resource Filters: It is executed following authorization and prior to the next request pipeline.
- Action Filters: They are executed prior to and following the execution of an action method Exception Filters: It is used to manage global exceptions and is executed when an unhandled exception is thrown.
- Result Filters: These are executed following the implementation of the action method and prior to and after the execution of action results.
There are Action Filters in.Net Core.
Action filters execute immediately before and after the execution of an action method. It can perform multiple operations, including modifying the passed arguments and the output.
How Do I Configure Action Filters?
Globally Tailored Action Based
The subsequent stages are identical for both
- Create a filter by extending a class that implements the IActionFilter interface.
- It will require you to implement these two procedures. OnActionExecuted and OnActionExecuting are event handlers.
- OnActionExecuted is executed after the method, while the other is executed before the method is invoked.
Global Action Filtering System
After creating this filter, you must add it to the controllers services container.
public class GlobalFilter: IActionFilter {
public void OnActionExecuted(ActionExecutedContext context) {
Console.WriteLine("Global Action Executed");
}
public void OnActionExecuting(ActionExecutingContext context) {
Console.WriteLine("Global Action is Executing");
}
}
public void ConfigureServices(IServiceCollection services) {
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_2);
services.AddHangfire(x =>
x.UseSqlServerStorage("Data Source=DJHC573\\MYSYSTEM;Initial Catalog=Hangfire-DB;Integrated Security=true;Max Pool Size = 200;"));
services.AddHangfireServer();
services.AddMvcCore(options => {
options.Filters.Add(new GlobalFilter());
});
}
That’s all you need to do, so this one will run whenever any action gets called.
Custom Action-Based Filter
Add your filter in Startup.cs, if you have multiple filters, add them in a similar fashion.
Add ServiceFilter(typeof(YourFilter)) at your controller level or action level, that’s all.
public class NewsletterFilter: IActionFilter {
public void OnActionExecuted(ActionExecutedContext context) {
Console.WriteLine("Action is Executed");
}
public void OnActionExecuting(ActionExecutingContext context) {
Console.WriteLine("Action is Executing");
}
}
public void ConfigureServices(IServiceCollection services) {
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_2);
//services.AddHangfire(x =>
//x.UseSqlServerStorage("Data Source=DJHC573\\MYSYSTEM;Initial Catalog=Hangfire-DB;Integrated Security=true;Max Pool Size = 200;"));
//services.AddHangfireServer();
services.AddMvcCore(options => {
options.Filters.Add(new GlobalFilter());
});
services.AddScoped < NewsletterFilter > ();
}
public class ValuesController: ControllerBase {
// GET api/values
[ServiceFilter(typeof (NewsletterFilter))]
[HttpGet]
public ActionResult < IEnumerable < string >> Get() {
return new string[] {
"value1",
"value2"
};
}
}
When I applied Global Action Filter and Newsletter (Custom Filters) on the same controller, it executed like this.
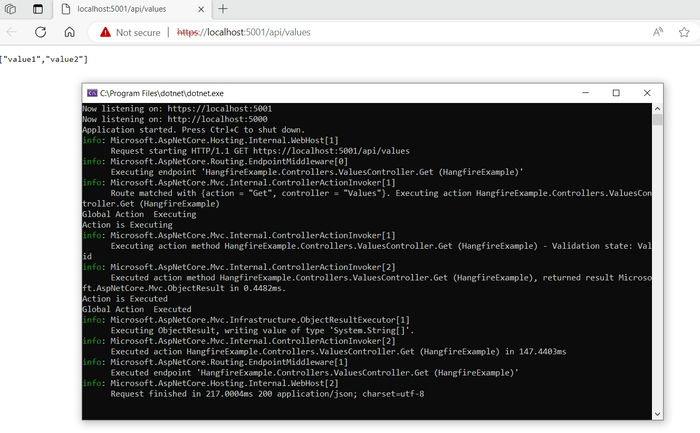
*If we want an asynchronous action filter, then we can inherit from IAsyncActionFilter instead of IActionFilter it has an extra method OnActionExecutionAsync which takes action context and delegate in parameters.
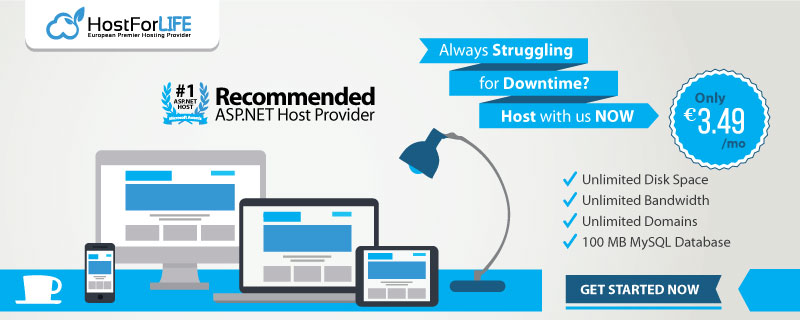