
October 27, 2014 09:33 by
Peter
A tooltip is a little pop-up window that seems when a user pauses the mouse pointer over a part, like over a Button. In this tutorial, I will show you how to disable the Tooltip in ASP.NET 4.5.2. Take a glance at the code below:
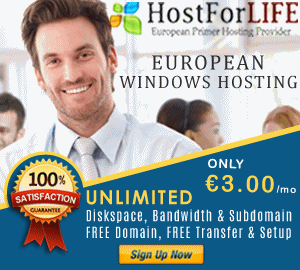
JavaScript
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script type="text/javascript">
function DisableToolTip() {
// Get the object
var obj = document.getElementById('LnkBtn');
// set the tool tip as empty
obj.title = "";
}
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:LinkButton runat="server" Text="Simple Link Button" ToolTip="Simple Button with Tool Tip" ID="LnkBtn" ClientIDMode="Static" ></asp:LinkButton>
<a href="#" id="lnkDisable" onclick="DisableToolTip();">Disable Tool Tip</a>
</form>
</body>
</html>

JQuery
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="Scripts/jquery-1.4.1.min.js" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#lnkDisable").click(function () { $('#LnkBtn').attr({'title':''}); });
});
</script>
</head>
<body>
<form id="form1" runat="server">
<asp:LinkButton runat="server" Text="Simple Link Button" ToolTip="Simple Button with Tool Tip" ID="LnkBtn" ClientIDMode="Static" ></asp:LinkButton>
<a href="#" id="lnkDisable">Disable Tool Tip</a>
</form>
</body>
</html>