
January 11, 2017 07:18 by
Peter
In this post, I will tell you about how to create multi step form in ASP.NET. Multiview will be used to display the content on the tap of Tab.
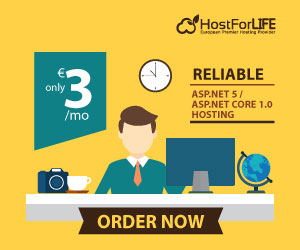
To show tab on the top, we will use Menu control. Thus, let's go and Add menu control from toolbox. I have given the Horizontal orientation, so that Tab will display horizontally.
<asp:Menu
ID="menuTabs"
Orientation="Horizontal"
Width="100%"
runat="server">
<Items>
<asp:MenuItem Text="Employee Info" Value="0" Selected="true"/>
<asp:MenuItem Text="Contact Info" Value="1" />
<asp:MenuItem Text="Salary Info" Value="2" />
</Items>
</asp:Menu>
To display the content for each tab, we will use Multiview control. I have given a property Selected="true" for Employee Info, so that when a page is launched, it will display the content of Employee Info.
<asp:MultiView ID="multiviewEmployee"
runat="server" ActiveViewIndex="0">
</asp:MultiView>
Next step is to add view inside Multiview. As I have three menu items, I need to add three views inside Multiview.
<asp:MultiView ID="multiviewEmployee"
runat="server" ActiveViewIndex="0">
<asp:View runat="server">
<div>
<%--To do--%>
</div>
</asp:View>
<asp:View runat="server">
<div>
<%--To do--%>
</div>
</asp:View>
<asp:View runat="server">
<div>
<%--To do--%>
</div>
</asp:View>
</asp:MultiView>
ActiveViewIndex= "0" will display first view after page is launched. Now, we will open corresponding view, when it is clicked on menu items. For it, we need to handle OnMenuItemClick event of Menu control. Hence, add it in your code and it will look, as mentioned below.
<asp:Menu
ID="menuTabs"
Orientation="Horizontal"
Width="100%"
runat="server"
OnMenuItemClick="menuTabs_MenuItemClick">
<Items>
<asp:MenuItem Text="Employee Info" Value="0" Selected="true"/>
<asp:MenuItem Text="Contact Info" Value="1" />
<asp:MenuItem Text="Salary Info" Value="2" />
</Items>
</asp:Menu>
In CS page, assign the value of menu item to multiview .
protected void menuTabs_MenuItemClick(object sender, MenuEventArgs e)
{
Menu menuTabs = sender as Menu;
MultiView multiTabs = this.FindControl("multiviewEmployee") as MultiView;
multiTabs.ActiveViewIndex = Int32.Parse(menuTabs.SelectedValue);
}
Now, it's none. Your tab structure is ready. Full code for ASPX is mentioned below.
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style>
.viewCSS {
border: solid 2px black;
padding: 20px;
}
#menuTabs a.static.selected {
border-bottom-color: red;
border-bottom-style: solid;
border-bottom-width: 3px;
color: red;
}
</style>
</head>
<body>
<form id="form1" runat="server" style="width: 100%">
<div style="width: 100%; margin-left: 20px; margin-top: 50px; margin-right: 20px;">
<asp:Menu
ID="menuTabs"
Orientation="Horizontal"
Width="100%"
runat="server"
OnMenuItemClick="menuTabs_MenuItemClick">
<Items>
<asp:MenuItem Text="Employee Info" Value="0" Selected="true" />
<asp:MenuItem Text="Contact Info" Value="1" />
<asp:MenuItem Text="Salary Info" Value="2" />
</Items>
</asp:Menu>
<asp:MultiView ID="multiviewEmployee"
runat="server" ActiveViewIndex="0">
<asp:View runat="server">
<div style="margin-top: 40px;">
<asp:Table runat="server" CssClass="viewCSS">
<asp:TableRow>
<asp:TableCell>
<asp:Label runat="server">First Name</asp:Label>
</asp:TableCell>
<asp:TableCell>
<asp:TextBox runat="server"></asp:TextBox>
</asp:TableCell>
</asp:TableRow>
<asp:TableRow>
<asp:TableCell>
<asp:Label runat="server">Last Name</asp:Label>
</asp:TableCell>
<asp:TableCell>
<asp:TextBox runat="server"></asp:TextBox>
</asp:TableCell>
</asp:TableRow>
</asp:Table>
</div>
</asp:View>
<asp:View runat="server">
<div style="margin-top: 40px;">
<asp:Table runat="server" CssClass="viewCSS">
<asp:TableRow>
<asp:TableCell>
<asp:Label runat="server">Address</asp:Label>
</asp:TableCell>
<asp:TableCell>
<asp:TextBox runat="server"></asp:TextBox>
</asp:TableCell>
</asp:TableRow>
<asp:TableRow>
<asp:TableCell>
<asp:Label runat="server">Mobile</asp:Label>
</asp:TableCell>
<asp:TableCell>
<asp:TextBox runat="server"></asp:TextBox>
</asp:TableCell>
</asp:TableRow>
</asp:Table>
</div>
</asp:View>
<asp:View runat="server">
<div style="margin-top: 40px;">
<asp:Table runat="server" CssClass="viewCSS">
<asp:TableRow>
<asp:TableCell>
<asp:Label runat="server">Hire Date</asp:Label>
</asp:TableCell>
<asp:TableCell>
<asp:TextBox runat="server"></asp:TextBox>
</asp:TableCell>
</asp:TableRow>
<asp:TableRow>
<asp:TableCell>
<asp:Label runat="server">Salary</asp:Label>
</asp:TableCell>
<asp:TableCell>
<asp:TextBox runat="server"></asp:TextBox>
</asp:TableCell>
</asp:TableRow>
</asp:Table>
</div>
</asp:View>
</asp:MultiView>
</div>
</form>
</body>
</html>
I Hope it works for you!
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
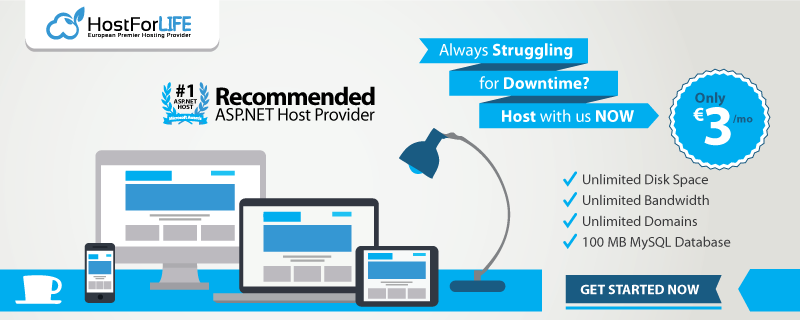