
October 9, 2018 09:07 by
Peter
Why Is Canopy Stabilization Layer Built on Top of Selenium?
One of the most crucial concepts of Canopy is reliability - when performing an action the framework tries, during the time span specified via elementTimeout or compareTimeout or pageTimeout, before failing. This improves the experience of writing tests.
Expressiveness
The syntax looks pretty self-explanatory:
"Bio should contain twitter link" &&& fun _ ->
url "https://github.com/peter"
".user-profile-bio" == "https://twitter.com/peter"
F#
In one of my previous articles, I have already expressed my opinion regarding power and expressiveness of F#.
Writing More Tests
To start, just create a console application, install nuget package canopy and create tests in Program.fs like below:
open canopy.configuration
open canopy.runner.classic
open System
open canopy.classic
//set path for chrome direver explicitly
chromeDir <- "C:\\"
start chrome
"Left bottom repostitory should be stationwalk.server" &&& fun _ ->
//visit the following url
url "https://github.com/peter"
//get 4th child of the following selector
let repo = nth 4 ".pinned-repo-item"
//get element with the following selector inside repo element
let firstRepoCaption = repo |> someElementWithin ".js-repo"
match firstRepoCaption with
| Some caption -> read caption == "stationwalk.server" //if found read element caption
//and compare it
| None _ -> failwith "Element not found" //if none element found throw an exception
"Left bottom repostitory should be stationwalk.client" &&& fun _ ->
url "https://github.com/peter"
let repo = nth 5 ".pinned-repo-item"
let firstRepoCaption = repo |> someElementWithin ".js-repo"
match firstRepoCaption with
| Some caption -> read caption == "stationwalk.client"
| None _ -> failwith "Element not found"
"Bio should contain twitter link" &&& fun _ ->
url "https://github.com/peter"
".user-profile-bio" == "https://twitter.com/peter"
run()
printfn "Press any key to exit..."
Console.ReadKey() |> ignore
quit()
Accessing IWebDriver
If you've ever written tests with Selenium using C#, you might be aware of IWebDriver interface which you still might use for some advanced configuration. For example, let's say we want to run our tests with a browser opened fullscreen. Then we can add the following function to our Program.fs file
let maximizeBrowser (browser : IWebDriver) =
browser.Manage().Window.Maximize()
Accessing IWebElement
Most of canopy's assertions, i.e., == accept as a parameter either a string which can be css or xpath selector or instance of IWebElement type which again might be already familiar to you if you've ever written selenium tests using C#. So let's say we want to upload something into file upload control.
let uploadFile fullFilePath =
(element "input[type='file']").SendKeys(fullFilePath)
Splitting Up Big File
Patterns which I've practiced to keep test project maintainable include extracting selectors into page modules and moving tests to separate files.
Let's revisit our github example by moving out selectors into the separate module:
module GithubProfilePage
let pinnedRepository = ".pinned-repo-item"
let bio = ".user-profile-bio"
Now we can reference them in the test which we'll move into a separate module too:
module GithubProfileTests
open canopy.runner.classic
open canopy.classic
let all() =
context "Github page tests"
"Left bottom repostitory should be staionwalk.server" &&& fun _ ->
url "https://github.com/peter"
let repo = nth 4 GithubProfilePage.pinnedRepository
let firstRepoCaption = repo |> someElementWithin ".js-repo"
match firstRepoCaption with
| Some caption -> read caption == "stationwalk.server"
| None _ -> failwith "Element not found"
"Right bottom repostitory should be staionwalk.client" &&& fun _ ->
url "https://github.com/peter"
let repo = nth 5 GithubProfilePage.pinnedRepository
let firstRepoCaption = repo |> someElementWithin ".js-repo"
match firstRepoCaption with
| Some caption -> read caption == "stationwalk.client"
| None _ -> failwith "Element not found"
"Bio should contain twitter link" &&& fun _ ->
url "https://github.com/peter"
GithubProfilePage.bio == "https://twitter.com/peter"
Our Program.fs will look like this:
open canopy.configuration
open canopy.runner.classic
open System
open canopy.classic
chromeDir <- "C:\\"
start chrome
GithubProfileTests.all()
run()
printfn "Press any key to exit..."
Console.ReadKey() |> ignore
quit()
Running Test in Parallel
Recently, Canopy had a major upgrade from 1.x to 2.x and one of the great new features is the ability to run tests in parallel.
Let's revisit our example by using this ability:
module GithubProfileTests
open canopy.parallell.functions
open canopy.types
open prunner
let all() =
"Left bottom repostitory should be stationwalk.server" &&& fun _ ->
let browser = start Chrome
url "https://github.com/peter" browser
let repo = nth 4 GithubProfilePage.pinnedRepository browser
let firstRepoCaption = someElementWithin ".js-repo" repo browser
match firstRepoCaption with
| Some caption -> equals (read caption browser) "stationwalk.server" browser
| None _ -> failwith "Element not found"
"Right bottom repostitory should be stationwalk.client" &&& fun _ ->
let browser = start Chrome
url "https://github.com/peter" browser
let repo = nth 5 GithubProfilePage.pinnedRepository browser
let firstRepoCaption = someElementWithin ".js-repo" repo browser
match firstRepoCaption with
| Some caption -> equals (read caption browser) "stationwalk.client" browser
| None _ -> failwith "Element not found"
"Bio should contain twitter link" &&& fun _ ->
let browser = start Chrome
url "https://github.com/peter" browser
equals GithubProfilePage.bio "https://twitter.com/peter" browser
The key trick to follow here is that each test operates now with its own copy of browser and assertions are now taken from open canopy.parallel.functions to accept browser as an argument.
Headless Testing
Testing in a headless browser seems to be the new black now. Although I don't share the sentiment, I still can assure you that testing in headless browsers is supported by Canopy. You can run your tests in headless Chrome as follows:
let browser = start ChromeHeadless
I hope this article has convinced you that Canopy is a robust and easy to use framework which can be used in building end-to-end testing layers of your application.
HostForLIFE.eu ASP.NET Core 2.2.1 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
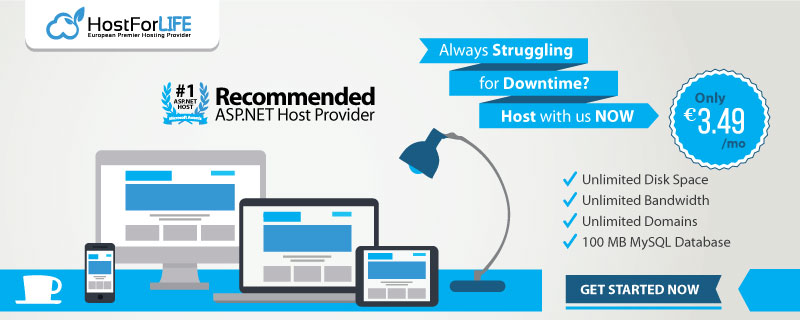