After submitting the download link, follow these steps to import the ADCaptcha.dll into an ASP.NET project:
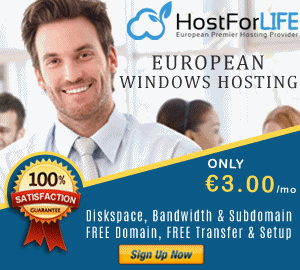
Provide a download link to the ADCaptcha.dll file on your website or any other platform where users can access it.
Include ADCaptcha.dll in the project: After downloading the ADCaptcha.dll, you must include it in the references section of your ASP.NET project.
- In the Solution Explorer of your project, right-click on the "References" node.
- Select "Add Reference."
- Locate the downloaded ADCaptcha.dll file by clicking the "Browse" option.
- Click "Add" to add the ADCaptcha.dll file to the project's references.
Import ADCaptcha Namespace: Import the necessary namespaces into the files where you want to use the ADCaptcha library. For example, if your ADCaptcha.dll has the namespace ADCaptcha, import it in the code files where you use the CAPTCHA functionalities:
using ADCaptcha; // Import the ADCaptcha namespace.
Utilize the ADCaptcha Library: Now that you have imported the ADCaptcha namespace, you can use the CAPTCHA functionalities provided by the ADCaptcha.dll in your code. For example:
using ADCaptcha;
// ... (other code)
// Generate a new CAPTCHA text and image.
string captchaText = CaptchaGenerator.GenerateRandomText(6, DifficultyMode.Medium);
byte[] captchaImageBytes = CaptchaGenerator.GenerateCaptchaImage(captchaText, 200, 60, 30, Color.White, Color.DarkBlue, DistortionTechnique.Warp, DistortionTechnique.NoiseLines);
// ... (other code)
By following these steps, you can successfully import the ADCaptcha.dll into your ASP.NET project and leverage its CAPTCHA generation and verification capabilities to secure your website from automated bots and spam.
How to generate a CAPTCHA image and verify user input
Below are example usages and sample code for the ADCAPTCHA DLL. We will demonstrate how to generate a CAPTCHA image in an ASP.NET web form and how to verify user input against the CAPTCHA text.
Generating and Displaying a CAPTCHA Image (ASP.NET Web Form)
In your ASP.NET web form (e.g., CaptchaPage.aspx), add an Image control to display the CAPTCHA image:
<asp:Image ID="CaptchaImage" runat="server" />
<asp:TextBox ID="UserInputTextBox" runat="server" CssClass="form-control mt-2"></asp:TextBox>
<asp:Button ID="SubmitButton" runat="server" Text="Submit" OnClick="SubmitButton_Click" CssClass="btn btn-info mt-2" />
<%--For Testing Purpose Only DLL By ASHOK DUDI--%>
<asp:Label Text="" ID="lblMsg" runat="server" />
In the code-behind file (CaptchaPage.aspx.cs), add the following code:
using ADCaptcha;
Add the below code to Page_Load event
if (!IsPostBack)
{
// Generate a new CAPTCHA text (You can also store this in session for verification later).
string captchaText = CaptchaGenerator.GenerateRandomText(6, DifficultyMode.Medium);
// Generate the CAPTCHA image and convert it to a base64 string.
byte[] captchaImageBytes = CaptchaGenerator.GenerateCaptchaImage(captchaText, 200, 60, 24, System.Drawing.Color.White, System.Drawing.Color.DarkBlue,DistortionTechnique.NoiseLines,DistortionTechnique.Swirl, DistortionTechnique.Warp);
// You can use anyone as required. Generate the CAPTCHA image and convert it to a base64 string.
//byte[] captchaImageBytes = CaptchaGenerator.GenerateCaptchaImage(captchaText, 200, 60);
string captchaImageBase64 = Convert.ToBase64String(captchaImageBytes);
// Set the CAPTCHA image source to the base64 string.
CaptchaImage.ImageUrl = "data:image/png;base64," + captchaImageBase64;
CaptchaImage.BorderColor = System.Drawing.Color.DarkBlue;
CaptchaImage.BorderWidth = 1;
// Store the CAPTCHA text in a session for verification during form submission.
Session["CaptchaText"] = captchaText;
}
To verify Captcha, use the below code on SubmitButton_Click event
protected void SubmitButton_Click(object sender, EventArgs e)
{
lblMsg.Text = "";
// Retrieve the stored CAPTCHA text from the session.
string captchaText = Session["CaptchaText"] as string;
// Retrieve the user's input from the TextBox.
string userInput = UserInputTextBox.Text;
// Verify the user's input against the CAPTCHA text (case-insensitive comparison by default).
bool isCaptchaValid = CaptchaVerifier.VerifyCaptcha(captchaText, userInput,false);
if (isCaptchaValid)
{
// CAPTCHA verification successful.
// Proceed with the form submission or any other action.
// ...
lblMsg.Text = "Success";
// Optionally, you can remove the CAPTCHA text from the session to prevent reuse of the same CAPTCHA.
Session.Remove("CaptchaText");
}
else
{
lblMsg.Text = "Failed";
// CAPTCHA verification failed.
// Show an error message to the user and ask them to try again.
// ...
}
}
Remember to modify the code and styling to match the structure and design of your unique ASP.NET project. Additionally, ensure that the session state in your ASP.NET application is appropriately configured to save and retrieve the CAPTC. Remember to alter the code and styling to reflect your individual ASP.NET project structure and design. Additionally, check that the session state in your ASP.NET application is appropriately configured to save and retrieve the CAPTCHA text for verification.Text from HA for verification.
HostForLIFE.eu ASP.NET 8 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
