In this tutorial, we will show you how to convert a password into complicated in ASP.NET. We will ise SHA1 or MD5 algorithm to work around for converting your password into complicated.
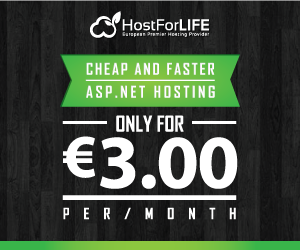
Follow these steps to make your password seems complicated in ASP.NET:
Step 1
Open Visual Studio and create an empty website. Give it a suitable name for example: [hashpass_demo].
Step 2
In Solution Explorer you will get your empty website, then add a Web Form by going like this:
- Right Click
- Add New Item, then Web Form. Name it hashpass_demo.aspx.
Step 3
Now mcreate some design for your application by going to hashpass_demo.aspx and try the code like this:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.style1
{
width: 258px;
}
.style2
{
width: 239px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<table style="width:100%;">
<tr>
<td class="style1">
Enter Your Username:</td>
<td class="style2">
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
</td>
<td>
<asp:Label ID="Label2" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td class="style1">
Enter Your Password:
</td>
<td class="style2">
<asp:TextBox ID="TextBox2" runat="server" TextMode="Password"></asp:TextBox>
</td>
<td>
<asp:Label ID="Label1" runat="server"></asp:Label>
</td>
</tr>
<tr>
<td class="style1">
</td>
<td class="style2">
</td>
<td>
</td>
</tr>
<tr>
<td class="style1">
</td>
<td class="style2">
<asp:Button ID="Button1" runat="server" onclick="Button1_Click" Text="Submit" />
</td>
<td>
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
Your design will look like following picture snippet:
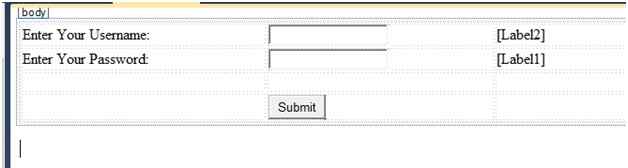
Step 4
Now it’s time for server side coding so that our application starts working. Open your hashpass_demo.aspx.cs file and code it like below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.Security;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
string securepass = FormsAuthentication.HashPasswordForStoringInConfigFile(TextBox2.Text, "MD5");
Label2.Text = "Your Username is-" + TextBox1.Text;
Label1.Text = "Your HashPassword is-" + securepass;
Label2.ForeColor = System.Drawing.Color.ForestGreen;
Label1.ForeColor = System.Drawing.Color.ForestGreen;
}
}
Here is the output:

Good luck!
HostForLIFE.eu ASP.NET 4.6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
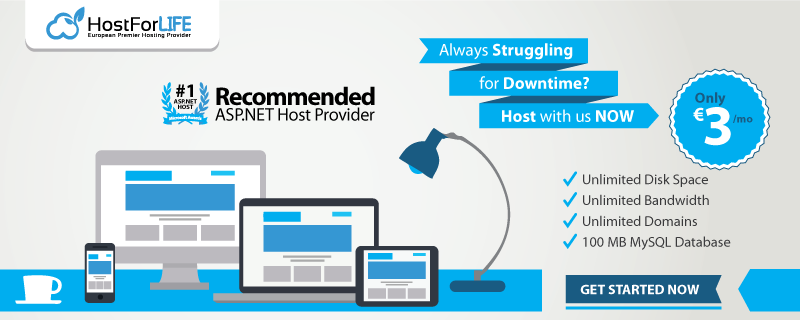