
April 22, 2024 08:38 by
Peter
Within software development, data extraction from PDF files is frequently required for a number of activities, including content indexing, information retrieval, and data analysis. Although ASP.NET Core 8 provides powerful tools for working with PDFs, there are times when developers would rather have other options due to project needs or flexibility concerns. In this post, we'll look at how to use the PdfSharpCore library to extract values from PDF files inside the.NET Core 8 environment without utilizing ASP.NET. We'll present you a detailed how-to and C# examples to show you how to complete this task successfully.
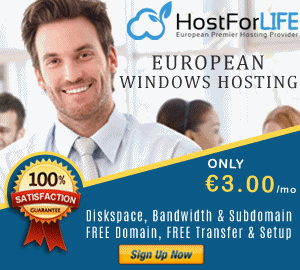
- Comprehending PdfSharpCore: PdfSharpCore is a well-liked.NET library designed for manipulating PDF documents. It offers tools for adding, editing, and removing material from PDF files. This tutorial will concentrate on using PdfSharpCore to extract text from PDF files.
- Installing PdfSharpCore: Installing the PdfSharpCore NuGet package is necessary before we can use PdfSharpCore in our.NET Core application. The.NET CLI or the NuGet Package Manager Console can be used for this.
Using the NuGet Package Manager Console
Install-Package PdfSharpCore
Using the .NET CLI
dotnet add package PdfSharpCore
Extracting Text from PDFs in C#: Now that we have PdfSharpCore installed, let's dive into how we can extract text from PDF files using C#.
using PdfSharpCore.Pdf;
using PdfSharpCore.Pdf.IO;
using System;
public class PdfTextExtractor
{
public static string ExtractTextFromPdf(string filePath)
{
using (PdfDocument document = PdfReader.Open(filePath, PdfDocumentOpenMode.Import))
{
string text = "";
foreach (PdfPage page in document.Pages)
{
text += page.GetText();
}
return text;
}
}
// Example usage:
public static void Main(string[] args)
{
string pdfText = ExtractTextFromPdf("sample.pdf");
Console.WriteLine(pdfText);
}
}
The extracted text is returned by the static method ExtractTextFromPdf, which we have implemented in this example. It accepts the file path of the PDF as input and returns the retrieved text. Within the procedure, we access the PDF file using PdfSharpCore, loop through its pages, and extract text from each one. The extracted text is then concatenated and sent back.
Conclusion
By leveraging PdfSharpCore in .NET Core 8, developers have access to a powerful and efficient tool for extracting text from PDF files. Without relying on ASP.NET, PdfSharpCore provides a straightforward solution for handling PDF documents within the .NET Core ecosystem. Whether you're building data processing pipelines, content management systems, or document parsing utilities, PdfSharpCore empowers developers to accomplish PDF manipulation tasks effectively and seamlessly.