The HttpContextAccessor class provides access to the present HTTP request and response context within ASP.NET Core Web API. It provides access to headers, cookies, query parameters, and user claims, among other aspects of the HTTP request and response.
Add Dependency to your program in Step 1.cs
Example Source Code builder.Services.AddSingletonIHttpContextAccessor, HttpContextAccessor>();
Utilizing HttpContextAccessor
In the example given below, the User is obtained, and then the company ID and user ID are obtained from the Auth Microservice.
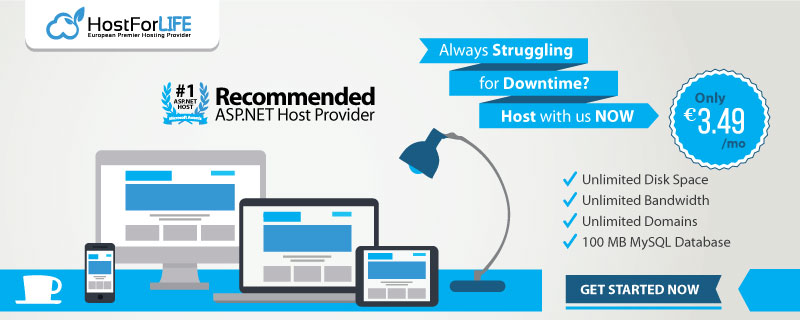
Example Source Code
builder.Services.AddSingletonIHttpContextAccessor, HttpContextAccessor>();
Utilizing HttpContextAccessor
In the example given below, the User is obtained, and then the company ID and user ID are obtained from the Auth Microservice.
Code Example
using AC_Projects_API_Domain_Layer.Models;
using AC_Projects_API_Repository_Layer.IRepository;
using AC_Projects_API_Service_Layer.IService;
using AutoMapper;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Claims;
using System.Text;
using System.Threading.Tasks;
namespace AC_Projects_API_Service_Layer.Services
{
public class ColumsService : ICustomService<Columns>
{
private readonly IRepository<Columns> _statusRepository;
public long LoggedInUserCompanyId;
public long LoggedInUserId;
private readonly IHttpContextAccessor _httpContextAccessor;
public ColumsService(
IRepository<Columns> statusRepository,
IMapper mapper,
IHttpContextAccessor httpContextAccessor
)
{
_statusRepository = statusRepository;
_httpContextAccessor = httpContextAccessor;
ClaimsIdentity user = (ClaimsIdentity)_httpContextAccessor.HttpContext.User.Identity;
LoggedInUserCompanyId = Convert.ToUInt32(user.FindFirst("CompanyId").Value.ToString());
LoggedInUserId = Convert.ToUInt32(user.FindFirst("UserId").Value.ToString());
}
public void Delete(Columns entity)
{
try
{
if (entity != null)
{
entity.ModifiedBy = LoggedInUserId;
entity.ModifiedDate = DateTime.Now;
_statusRepository.Delete(entity);
_statusRepository.SaveChanges();
}
}
catch (Exception)
{
throw;
}
}
public Columns Get(long Id)
{
try
{
var obj = _statusRepository.Get(Id);
if (obj != null)
{
return obj;
}
else
{
return null;
}
}
catch (Exception)
{
throw;
}
}
public IEnumerable<Columns> GetAll()
{
try
{
var obj = _statusRepository.GetAll();
if (obj != null)
{
return obj;
}
else
{
return null;
}
}
catch (Exception)
{
throw;
}
}
public Columns Insert(Columns entity)
{
try
{
if (entity != null)
{
entity.CreatedBy = LoggedInUserId;
entity.CreatedDate = DateTime.Now;
entity.CompanyId = LoggedInUserCompanyId;
_statusRepository.Insert(entity);
_statusRepository.SaveChanges();
return entity;
}
return null;
}
catch (Exception)
{
throw;
}
}
public void Remove(Columns entity)
{
try
{
if (entity != null)
{
_statusRepository.Remove(entity);
_statusRepository.SaveChanges();
}
}
catch (Exception)
{
throw;
}
}
public void Update(Columns entity)
{
try
{
if (entity != null)
{
entity.ModifiedBy = LoggedInUserId;
entity.ModifiedDate = DateTime.Now;
_statusRepository.Update(entity);
_statusRepository.SaveChanges();
}
}
catch (Exception)
{
throw;
}
}
}
}
Why'd we Use HttpContextAccessor?
For the following reasons, the `HttpContextAccessor` is frequently utilized in ASP.NET Core Web API applications:
Accessing HTTP Request and Response
Access to the current HTTP request and response context is the main goal of the `HttpContextAccessor` component. You can use it to get details about incoming requests, including headers, cookies, query parameters, and user claims. By gaining access to the "Response" property, you can also alter the HTTP response.
Authentication and Authorization
You can gain access to authentication-related data via the `HttpContextAccessor`, including the claims, identity, and roles of an authenticated user. This can be used to implement authorization checks inside of your application, figure out the permissions of the current user, or alter behavior based on authentication status.
Logging and Diagnostics
You can record information relevant to the current HTTP request using the `HttpContextAccessor' To assist with debugging or monitoring, you can use it to record request-specific information, such as the URL, headers, or user information. It enables you to effectively detect problems by correlating log entries with particular requests.
Custom Middleware and Filters
You can design action filters or middleware components in ASP.NET Core that need access to the HTTP context. You can access request-specific data and adjust the request or response pipeline by using the `HttpContextAccessor`, which enables you to inject the current context into your custom components.
Integration with External Services
To carry out specific tasks, some third-party services or libraries can need access to the HTTP context. These external components or services can interact with the current request and response by utilizing the "HttpContextAccessor" to give them the necessary context.
Although the "HttpContextAccessor" offers practical access to the HTTP context, it should only be used sparingly. By exposing the HTTP context too broadly in your application, you risk creating security holes or adding dependencies that make it harder to maintain your code. The use of "HttpContextAccessor" in your application should, therefore, be carefully considered in terms of its scope and objectives.
The ASP.NET Core Web API's `ttpContextAccessor` gives users access to the current HTTP request and response context. It enables you to carry out actions based on authentication and authorization, alter the response, and retrieve information about the request. It is frequently employed for logging, diagnostics, developing unique middleware, interfacing with outside services, and other purposes. It should be utilized cautiously, taking into account security issues and keeping a clean codebase.