
February 28, 2024 07:16 by
Peter
ASP.NET Core is a sophisticated framework for developing online APIs that enables developers to build strong and scalable applications. One of the most important aspects of current web development is the use of third-party APIs, which give access to external services and data.
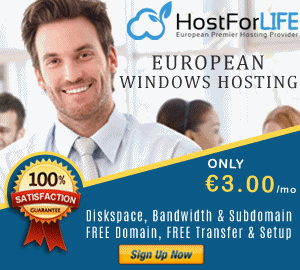
Create a Model
using System.Text.Json.Serialization;
namespace _1_3rdAPIIntegrationInAspNetCoreWebAPI.Models
{
public class Product
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
public double DiscountPercentage { get; set; }
public double Rating { get; set; }
public int Stock { get; set; }
public string Brand { get; set; }
public string Category { get; set; }
public string Thumbnail { get; set; }
public List<string> Images { get; set; }
}
}
Here's a breakdown of each property.
- ID: An integer representing the unique identifier of the product.
- Title: A string representing the title or name of the product.
- Description: A string representing the description or details of the product.
- Price: A decimal representing the price of the product.
- DiscountPercentage: A double representing the discount percentage applied to the product.
- Rating: A double representing the rating of the product (e.g., star rating).
- Stock: An integer representing the available quantity of the product in stock.
- Brand: A string representing the brand or manufacturer of the product.
- Category: A string representing the category or type of the product.
- Thumbnail: A string representing the URL or path to the thumbnail image of the product.
- Images: A list of strings representing the URLs or paths to additional images of the product.
Create the Interface for the Product Service
using _1_3rdAPIIntegrationInAspNetCoreWebAPI.Models;
namespace _1_3rdAPIIntegrationInAspNetCoreWebAPI.Interfaces
{
public interface IProducts
{
Task<List<ProductsResponse>> GetProductsAsync();
}
}
GetProductsAsync: This function returns a Task<List<ProductsResponse>>. It indicates that implementing classes will enable the asynchronous retrieval of a list of products. The List<ProductsResponse> contains product responses, including IDs, titles, descriptions, and pricing. The method is asynchronous, as shown by the Task return type, which means that it can be anticipated for asynchronous execution.
Create the service for the products
using _1_3rdAPIIntegrationInAspNetCoreWebAPI.Interfaces;
using _1_3rdAPIIntegrationInAspNetCoreWebAPI.Models;
using System.Net;
using System.Text.Json;
namespace _1_3rdAPIIntegrationInAspNetCoreWebAPI.Services
{
public class ProductService : IProducts
{
private static readonly HttpClient httpClient;
static ProductService()
{
httpClient = new HttpClient()
{
BaseAddress = new Uri("https://dummyjson.com/")
};
}
public async Task<List<ProductsResponse>> GetProductsAsync()
{
try
{
var url = string.Format("products");
var result = new List<ProductsResponse>();
var response = await httpClient.GetAsync(url);
if (response.IsSuccessStatusCode)
{
var stringResponse = await response.Content.ReadAsStringAsync();
var productsResponse = JsonSerializer.Deserialize<ProductsResponse>(stringResponse, new JsonSerializerOptions()
{
PropertyNamingPolicy = JsonNamingPolicy.CamelCase
});
// Add the deserialized ProductsResponse to the result list
result.Add(productsResponse);
}
else
{
if (response.StatusCode == HttpStatusCode.NotFound)
{
throw new Exception("Products not found.");
}
else
{
throw new Exception("Failed to fetch data from the server. Status code: " + response.StatusCode);
}
}
return result;
}
catch (HttpRequestException ex)
{
throw new Exception("HTTP request failed: " + ex.Message);
}
catch (JsonException ex)
{
throw new Exception("JSON deserialization failed: " + ex.Message);
}
catch (Exception ex)
{
throw new Exception("An unexpected error occurred: " + ex.Message);
}
}
}
}
Create the Controller for the Products
using _1_3rdAPIIntegrationInAspNetCoreWebAPI.Interfaces;
using _1_3rdAPIIntegrationInAspNetCoreWebAPI.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
namespace _1_3rdAPIIntegrationInAspNetCoreWebAPI.Controllers
{
[Route("api/[controller]/[action]")]
[ApiController]
public class ProductsController : ControllerBase
{
private readonly IProducts _productService;
public ProductsController(IProducts productService)
{
_productService = productService;
}
[HttpGet]
public async Task<IEnumerable<ProductsResponse>> GetProducts()
{
return await _productService.GetProductsAsync();
}
}
}
Register the Services in IOC Container
using _1_3rdAPIIntegrationInAspNetCoreWebAPI.Interfaces;
using _1_3rdAPIIntegrationInAspNetCoreWebAPI.Services;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddSingleton<IProducts, ProductService>();
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
Output
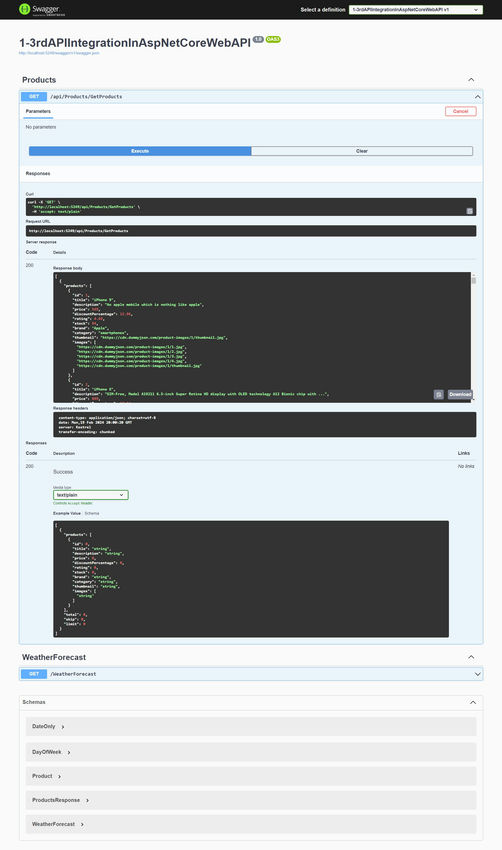