Logs are a crucial component of application development since they let you keep an eye on and diagnose issues and defects in your program. Developers love Serilog because it offers organized logging, which makes it easy to find and fix problems. A diagnostic logging library called Serilog was created especially for.NET applications. This method of logging application events, failures, and other pertinent data is straightforward, adaptable, and effective. Support for structured logging, which enables developers to log rich, structured data rather than just plain text messages, is one of Serilog's main advantages.
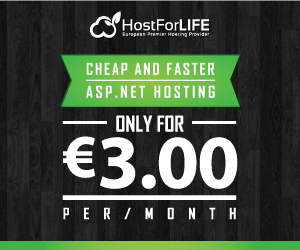
This post will teach us how to use Serilog with sink AppInsights for application logging in an ASP.NET Core Web API project. Let's now proceed to using Verilog to develop application logging using stages.
Step 1: If your project requires the use of AppInsights for Serilog logging, create a new ASP.NET Core Web API application (.NET8) or utilize an already-existing one.

Give the project name, select the location of the project, and Click on Next.

Select project framework: .NET 8, as depicted below.

Step 2. Install the following packages in your project from the NuGet package manager.
- Serilog
- Serilog.AspNetCore
- Serilog.Sinks.File
- Serilog.Sinks.ApplicationInsights
Step 3. After the installation of the necessary packages, we will need to configure Serilog in the appsetting.json file. Implementing logging with Verilog in local files and Appinsights is almost similar. However, there are some changes, such as in Using, Write To, and so on, which you can see in the below appsetting.json file.
Note. You need to have an Azure subscription and have already created application insights for this configuration to work.
"Serilog": {
"Using": [
"Serilog.Sinks.ApplicationInsights"
],
"MinimumLevel": {
"Default": "Information",
"Override": {
"Microsoft": "Warning",
"System": "Warning"
}
},
"WriteTo": [
{
"Name": "ApplicationInsights",
"Args": {
"connectionString": "",
"telemetryConverter": "Serilog.Sinks.ApplicationInsights.TelemetryConverters.TraceTelemetryConverter, Serilog.Sinks.ApplicationInsights"
}
}
],
"Enrich": [ "FromLogContext" ],
"Properties": {
"Application": "Connect.IMS"
}
},
If you want to log in to the local file, you can refer to the previous article here.
Step 4. In the Program.cs, we will add the Serilog configuration
builder.Host.UseSerilog((context, configuration) =>
configuration.ReadFrom.Configuration(context.Configuration));
Step 5. Then, above the App.Run() write Serilog middleware.
app.UseSerilogRequestLogging();
Step 6. Then, we can now log in to any C# class.
Below is an example of logging in TestController.
using Microsoft.AspNetCore.Mvc;
namespace SampleLogger.Controllers;
[ApiController]
[Route("[controller]")]
public class TestController : ControllerBase
{
private readonly ILogger<TestController> _logger;
public TestController(ILogger<TestController> logger)
{
_logger = logger;
}
[HttpGet]
[ProducesResponseType(StatusCodes.Status200OK)]
public IActionResult Get()
{
_logger.LogInformation("Test Controller called!");
return Ok();
}
}
Now, we can run the application and check the logs in application insights.
Conclusion
By integrating Serilog to produce more thorough and useful log data, developers can enhance debugging, monitoring, and system maintenance. Because of its structured logging features, Serilog is crucial for contemporary applications as they facilitate log analysis and visualization. The traceability, dependability, and general performance of your application can all be greatly enhanced by using Serilog. This tutorial taught us how to use Application Insights and Serilog to log apps. I hope it's useful to you.