
August 5, 2024 10:42 by
Peter
Unique identifying codes that ensure uniqueness are referred to as GUIDs (Globally Unique Identifier) and UIDs (Universally Unique Identifiers). They aren't precisely the same, though. In the realm of programming, the term UUID is used widely across many platforms, languages, and environments. Multiple iterations of the UUID standard are used to create distinct IDs. In the Microsoft ecosystem, UUIDs are referred to as GUIDs when they are utilized. This is a result of Microsoft using the UUID version 4 definition. Thus, GUID is included within the more general name UUID.
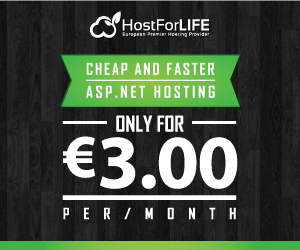
Why do we use GUID?
The most common use case for GUIDs is to identify unique records, components, or objects across different systems and databases. GUIDs can be used to identify unique records in databases, COM components (in the past), message identifiers in message queuing systems, session IDs and transaction IDs for session management, token identifiers in security and authentication scenarios, and so on.
Current Scenario
In C#, generating GUID is very simple, as shown below.
Guid g = Guid.NewGuid();
Console.WriteLine(g);
Problem
Although we have a guarantee that the GUID generated using the above code will always be unique, there are several use cases where this can be problematic.
Let’s create five different GUIDs.
Console.WriteLine(Guid.NewGuid());
Console.WriteLine(Guid.NewGuid());
Console.WriteLine(Guid.NewGuid());
Console.WriteLine(Guid.NewGuid());
Console.WriteLine(Guid.NewGuid());
Output
d61f4b57-31e9-4158-86a7-104c3cb16875
e7120f4f-bce1-488b-bdb3-4738793525bd
021baa3e-bf52-4d2e-8303-e1bdddea786b
c2e0c9f8-f04d-4d91-928e-cad5aca5b83f
96f8ae8c-808b-4fc1-a9b8-8e3066acdc50
As you can see from the above output, we cannot identify the order of GUIDs. This means we cannot sort on a field that stores GUIDs. This is essential if we store IDs in a database table and need to retrieve them in sorted order.
New Approach
The new approach is to use version 7 of UUID (instead of version 4) to generate GUIDs. Why version 7? Let’s discuss. If you refer to the original documentation of UUID Version 7, you will notice the description saying that it’s a time-ordered value field derived from the widely implemented and well-known Unix Epoch timestamp source, the number of milliseconds since midnight 1 Jan 1970 UTC, leap seconds excluded. Generally, UUIDv7 has improved entropy characteristics over UUIDv1 (Section 5.1) or UUIDv6 (Section 5.6).
The GUIDs have 5 parts (as you can see from the above example output). For UUID version 7, the first 48 bits (out of 128) will represent the Unix timestamp.
Basically, the IDs generated will be in order of time they generated. As a result, we will be able to sort columns storing GUIDs.
Console.WriteLine(Guid.CreateVersion7());
Console.WriteLine(Guid.CreateVersion7());
Console.WriteLine(Guid.CreateVersion7());
Console.WriteLine(Guid.CreateVersion7());
Console.WriteLine(Guid.CreateVersion7());
Output
5dad1927-6f23-4a07-a033-9dadae005ff7
5dad1927-6f23-4fe7-bfac-1f4dbbcd63ce
5dad1927-6f23-48ba-9044-99ba700770d5
5dad1927-6f23-481f-aa80-bbb32c54ec1c
5dad1927-6f23-4f8f-b639-bdff99b44b55
As you can see from the above output, the first few bits (“5dad1927–6f23”) are common, this is because they were created with the same timestamp. In fact, there is an overload of CreateVersion7 which can take the timestamp provider to control the timestamp.
var guid7 = Guid.CreateVersion7(timeProvider.GetUtcNow());
The advantages of using our own timestamp provider include the ability to provide past or future timestamps and using a DI container to provide our own fake provider.
An additional advantage is that we can always extract the date/time from the GUID if needed.
However, please note that from a performance perspective, version 7 takes longer than the existing version. So, if you are creating a large number of GUIDs, it's best to use the existing code and create another column for sorting.
Conclusion
We learned that GUID is an implementation of a specific version of UUID and is very specific to the Microsoft ecosystem. Further, starting from .NET 9, you can use GUIDs that can be sorted and created using a timestamp provider.