
August 22, 2024 06:50 by
Peter
In order to improve and customize user experiences, digital content authors and website managers must have a thorough understanding of how consumers engage with published information. Acknowledging the significance of engagement metrics, it was imperative to put in place a system that tracks and shows the number of page views for every post in real time.
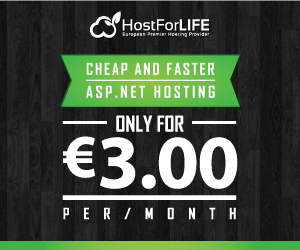
The requirement for this functionality stemmed from several key objectives.
- Immediate Feedback: Authors and administrators wanted immediate feedback on the performance of newly published articles.
- User Engagement: Displaying pageview counts can increase transparency and user engagement, as readers can see how popular an article is, potentially influencing their interactions (like comments and shares).
- Content Strategy Optimization: Real-time data helps in quickly identifying trends and reader preferences, enabling quicker adjustments to content strategy.
- Enhanced User Experience: Real-time updates contribute to a dynamic and interactive user experience, making the website feel more alive and responsive.
Putting such a feature into practice requires a number of contemporary tools and techniques. We'll use Entity Framework Core for database operations,.NET Core for server-side logic, and SignalR for real-time web functionality in this tutorial. Their strong performance, scalability, and broad community and Microsoft support are the main factors influencing these decisions.
Tools and Technologies
- .NET Core: A versatile platform for building internet-connected applications, such as web apps and services.
- Entity Framework Core: An object-database mapper that enables .NET developers to work with a database using .NET objects, eliminating the need for most data-access code.
- SignalR: A library for ASP.NET developers that simplifies the process of adding real-time web functionality to applications.
- SQL Database: Utilized to store and retrieve pageview data and article content efficiently.
Implementation Overview
To achieve real-time pageview tracking, we will implement the step-by-step example.
Model Setup: Define a data model for articles that includes a pageview count.
Database Configuration: Set up Entity Framework Core with SQL Server to manage data persistence.
SignalR Integration: Implement a SignalR hub to facilitate the real-time broadcasting of pageview counts to all connected clients.
Incrementing Views: Modify the server-side logic to increment pageview counts upon article access.
Client-Side Updates: Use SignalR on the client side to update the pageview count in real time as users visit the page.
Phase-by-Step Execution
This is how we can put real-time pageview tracking into practice.
Step 1. Define the Article Model
First, add a property to track page visits in the updated model.
public class Article
{
public int Id { get; set; }
public string Title { get; set; }
public string Content { get; set; }
public int PageViews { get; set; } // Tracks the number of views
}
Step 2. Configure Entity Framework Core
Configure Entity Framework Core in the Startup.cs to include our database context with SQL Server.
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddControllersWithViews();
services.AddSignalR();
}
Step 3. Implement SignalR Hub
Create a SignalR hub that manages the broadcasting of pageview updates to connected clients.
public class PageViewHub : Hub
{
public async Task UpdatePageViewCount(int articleId, int pageViews)
{
await Clients.All.SendAsync("ReceivePageViewUpdate", articleId, pageViews);
}
}
Step 4. Increment Pageviews in Controller
Modify the controller to increment the pageview count each time an article is viewed and notify clients using SignalR.
public async Task<IActionResult> ViewArticle(int id)
{
var article = await _context.Articles.FindAsync(id);
if (article == null)
{
return NotFound();
}
article.PageViews++;
_context.Update(article);
await _context.SaveChangesAsync();
await _hubContext.Clients.All.SendAsync("ReceivePageViewUpdate", article.Id, article.PageViews);
return View(article);
}
Step 5. Update Client-Side Code
On the client side, use SignalR JavaScript client to update the pageview count in real time.
@section Scripts {
<script src="~/lib/signalr/signalr.min.js"></script>
<script>
var connection = new signalR.HubConnectionBuilder().withUrl("/pageViewHub").build();
connection.on("ReceivePageViewUpdate", function (articleId, pageViews) {
var pageViewElement = document.getElementById("page-views-" + articleId);
if (pageViewElement) {
pageViewElement.innerText = pageViews + " Views";
}
});
connection.start().catch(function (err) {
return console.error(err.toString());
});
</script>
}
Conclusion
Now that real-time pageview monitoring is included, the platform can provide a more responsive and dynamic user experience. This feature improves user engagement while also offering insightful data that aids in the development of successful content initiatives. By utilizing contemporary technologies such as SignalR and.NET Core, we can maintain our status as a community-driven website that values and reacts to user interactions.