Cross-platform framework.NET MAUI enables developers to create native mobile and desktop applications using C# and XAML. It facilitates the creation of apps that operate seamlessly on Android, iOS, macOS, and Windows using a single codebase. This open-source platform is an evolution of Xamarin Forms, extending its applicability to desktop scenarios and enhancing UI controls for enhanced performance and extensibility.
Using.NET MAUI, you can create applications that run on multiple platforms, including Android, iOS, MacOS, and Windows, from a single codebase.
.NET MAUI enables efficient cross-platform development, eliminating the need for distinct codebases for each platform and simplifying application maintenance and updates.
.NET MAUI saves time and effort and simplifies application maintenance. Visual Studio 2022 supports dotnet 7 and.Net Maui application development
Cross-platform framework.NET MAUI enables developers to create native mobile and desktop applications using C# and XAML. It facilitates the creation of apps that operate seamlessly on Android, iOS, macOS, and Windows using a single codebase. This open-source platform is an evolution of Xamarin Forms, extending its applicability to desktop scenarios and enhancing UI controls for enhanced performance and extensibility.
This article demonstrates how to implement a File Picker in a.NET MAUI application.
Project Setup
To create a new project, launch Visual Studio 2022 and select Create a new project in the start window.
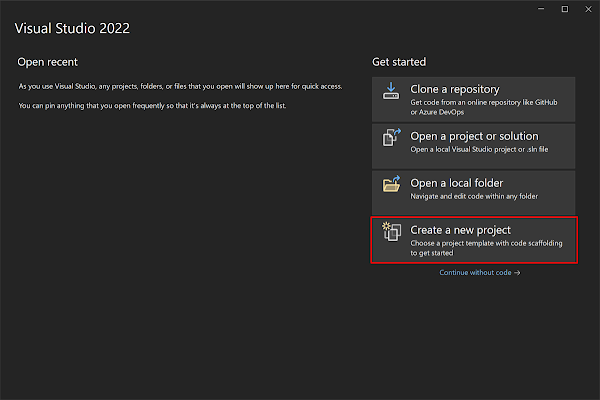
In the Create a new project window, select MAUI in the All project types drop-down, select the .NET MAUI App template, and click the Next button:
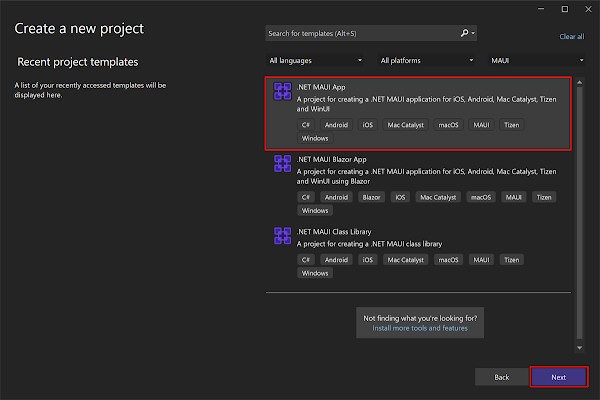
Give your project a name, pick an appropriate location for it, then click the Next button in the Configure your new project window:
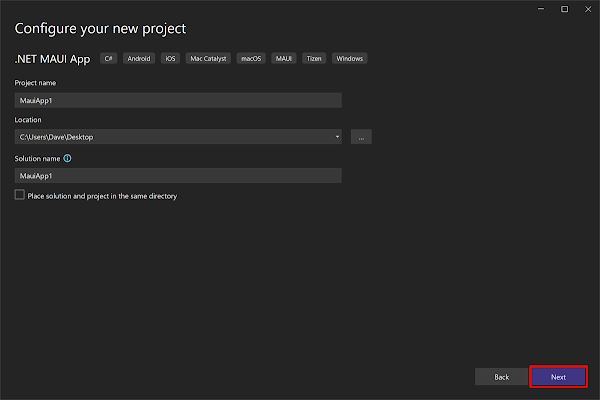
In the Additional information window, click the Create button:

Implementation
As a first point, we need to implement the screen design as per our requirements. In this tutorial, we will use 3 controls - button, image, and label like in the following code block.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiFilePicker.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30"
VerticalOptions="Start">
<Button
x:Name="ImageSelect"
Text="Select Barcode"
SemanticProperties.Hint="Select Image"
Clicked="SelectBarcode"
HorizontalOptions="Center" />
<Label x:Name="outputText"
Padding="10"/>
<Image
x:Name="barcodeImage"
SemanticProperties.Description="Selected Barcode"
HeightRequest="200"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
Here,
Button - used to select images from the device.
Image - used to display the selected image.
Label - used to display the file path once the image is selected through the file picker.
Functional part
Add a click event for the button to select the image like below.
private async void SelectBarcode(object sender, EventArgs e)
{
var images = await FilePicker.Default.PickAsync(new PickOptions
{
PickerTitle = "Pick Barcode/QR Code Image",
FileTypes = FilePickerFileType.Images
});
var imageSource = images.FullPath.ToString();
barcodeImage.Source = imageSource;
outputText.Text = imageSource;
}
Here,
FileTypes: The default file type available for selection in the FilePicker are FilePickerFileType.Images, FilePickerFileType.Png, and FilePickerFileType.Videos. However, if you need to specify custom file types for a specific platform, you can create an instance of the FilePickerFileType class. This allows you to define the desired file types according to your requirements.
PickerTitle: The PickOptions.PickerTitle is presented to the user and its behavior varies across different platforms.
Full Code
namespace MauiFilePicker;
public partial class MainPage : ContentPage
{
int count = 0;
public MainPage()
{
InitializeComponent();
}
private async void SelectBarcode(object sender, EventArgs e)
{
var images = await FilePicker.Default.PickAsync(new PickOptions
{
PickerTitle = "Pick Barcode/QR Code Image",
FileTypes = FilePickerFileType.Images
});
var imageSource = images.FullPath.ToString();
barcodeImage.Source = imageSource;
outputText.Text = imageSource;
}
}
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiFilePicker.MainPage">
<ScrollView>
<VerticalStackLayout
Spacing="25"
Padding="30"
VerticalOptions="Start">
<Button
x:Name="ImageSelect"
Text="Select Barcode"
SemanticProperties.Hint="Select Image"
Clicked="SelectBarcode"
HorizontalOptions="Center" />
<Label x:Name="outputText"
Padding="10"/>
<Image
x:Name="barcodeImage"
SemanticProperties.Description="Selected Barcode"
HeightRequest="200"
HorizontalOptions="Center" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
Demo
Android
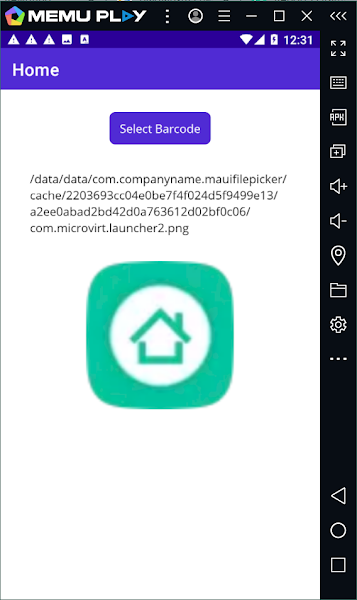
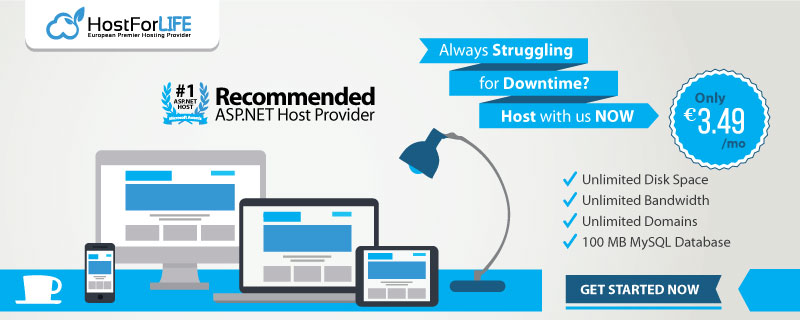