ASP.NET Core is a versatile and robust web application framework. Middleware is one of its core characteristics, and it plays a critical role in processing requests and responses as they travel through your application. This post will explain what middleware is, how it works, and why it is necessary for developing powerful online applications in ASP.NET Core.
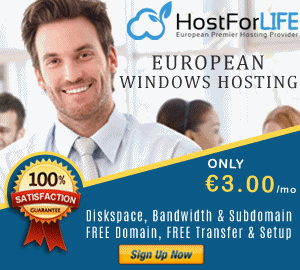
ASP.NET Core Middleware
In ASP.NET Core, middleware is a collection of components that are added to the application's request processing pipeline. Each middleware component is responsible for processing an incoming request or an outgoing answer. Middleware components can be added, removed, or reordered, allowing developers to tailor the request-handling pipeline to the specific demands of their application.
Middleware can handle a wide range of responsibilities, including authentication, routing, caching, logging, and more. It functions similarly to a chain of building blocks, with each block performing a specified operation on the request or response before passing it on to the next middleware in the pipeline.
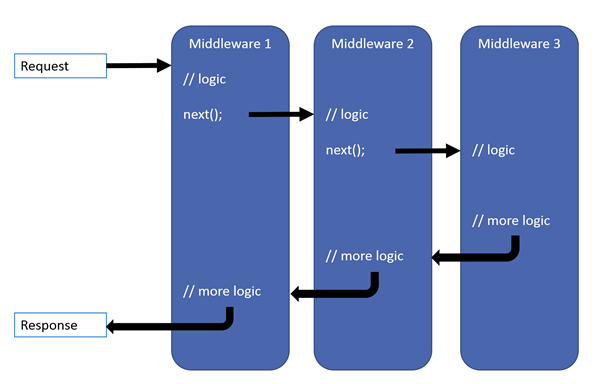
The Middleware Anatomy
In ASP.NET Core, middleware components are often implemented as classes with a specified signature. They accept an HTTP context containing information about the incoming request and allow them to modify the answer. This is the fundamental structure of middleware.
public class MyMiddleware
{
private readonly RequestDelegate _next;
public MyMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context)
{
// Do something before the next middleware
// ...
await _next(context); // Call the next middleware in the pipeline
// Do something after the next middleware
// ...
}
}
In this case, RequestDelegate represents the next middleware in the pipeline, and the InvokeAsync method provides the logic that will run before and after the next middleware.
In Use Middleware
Consider the following example, in which we wish to track the path of each incoming request.
public class LoggingMiddleware
{
private readonly RequestDelegate _next;
public LoggingMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context)
{
// Log the request path
Console.WriteLine($"Request to: {context.Request.Path}");
await _next(context); // Call the next middleware in the pipeline
}
}
We can add this middleware to the pipeline in the Startup.cs file.
public void Configure(IApplicationBuilder app)
{
app.UseMiddleware<LoggingMiddleware>();
// Other middleware and configuration...
}
Now, every incoming request's path will be logged before it proceeds through the pipeline.
Ordering Middleware
The order in which middleware components are added to the pipeline matters. Middleware is executed in the order it's added, from top to bottom. For example, if you want to perform authentication before routing, you should add authentication middleware before routing middleware.
public void Configure(IApplicationBuilder app)
{
app.UseAuthentication(); // Add authentication middleware
app.UseRouting(); // Add routing middleware
// Other middleware and configuration...
}
Middleware is a fundamental concept in ASP.NET Core, allowing you to modularize and customize request and response processing in your web applications. By understanding how middleware works and how to leverage it effectively, you can build robust and flexible web applications that meet your specific requirements. Whether you're handling authentication, logging, or any other aspect of request processing, middleware is a powerful tool in your ASP.NET Core toolbox.