
October 24, 2014 06:42 by
Peter
Today, I want to show you How to use jQuery Ajax in ASP.NET 4.5. jQuery permits you to call server-side ASP.NET methods from the client side with none PostBack. truly it's an Ajax call to the server however it permits us to decision call or function defined server-side. The code snippet below describes the syntax of the call.
$.ajax({
type: "POST",
url: "CS.aspx/MethodName",
data: '{name: "' + $("#<%=txtUserName.ClientID%>")[0].value + '" }',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
failure: function (response) {
alert(response.d);
}
});
HTML Markup
<div>
Your Name :
<asp:textbox id="txtUserName" runat="server"></asp:textbox>
<input id="btnGetTime" type="button" value="Show Current Time" onclick="ShowCurrentTime()" />
</div>
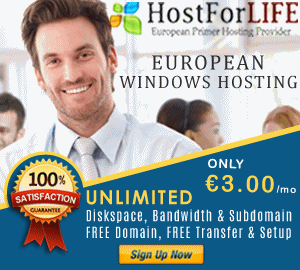
As you can see in the preceding I have added a TextBox when the user enters his name and a TML button that calls a JavaScript method to get the Current Time.
Methods used on Client Side
<script src="scripts/jquery-1.3.2.min.js" type="text/javascript"></script>
<script type="text/javascript">
function ShowCurrentTime() {
$.ajax({
type: "POST",
url: "CS.aspx/GetCurrentTime",
data: '{name: "' + $("#<%=txtUserName.ClientID%>")[0].value + '" }',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: OnSuccess,
failure: function (response) {
alert(response.d);
}
});
}
function OnSuccess(response) {
alert(response.d);
}
</script>
That ShowCurrentTime method above makes an AJAX call to the server. That method will executes the GetCurrentTime and accepts the username then returns a string value. This is the Server-Side Methods
C#
[System.Web.Services.WebMethod]
public static string GetCurrentTime(string name)
{
return "Hello " + name + Environment.NewLine + "The Current Time is: "
+ DateTime.Now.ToString();
}
VB.Net
<System.Web.Services.WebMethod()> _
ublic Shared Function GetCurrentTime(ByVal name As String) As String
Return "Hello " & name & Environment.NewLine & "The Current Time is: " & _
DateTime.Now.ToString()
End Function
The preceding method merely returns a acknowledgment message to the user along side the current server time. a vital factor to notice is that the method is declared as static (C#) or Shared (VB.Net) and also it's declared as a web method since unless you are doing this you will not be ready to decision the methods.