
November 24, 2014 06:35 by
Peter
In this tutorial, I will tell you how to use NServiceBus with ASP.NET SignalR. In detail right listed below what I'm planning to do is periodically publish an event utilizing NServiceBus. I will be able to use a ASP.NET MVC application as my front end and there I will be able to use a an additional NServiceBus client and that is liable for capturing event broadcasted from the NServiceBus publisher. Then working with ASP.NET SignalR, I will certainly be updating the UI with others captured events content.
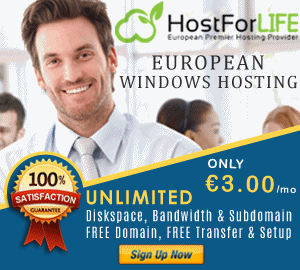
First, Add a new ASP. NET MVC application to the solution.
Currently I'm running NServiceBus. Host and Microsoft ASP. NET SignalR nuget packages on ASP. NET MVC project using Package Manager Console. NServiceBus. Host can add the EndpointConfig. cs towards the project and I'm keeping as it's. Currently I'm making a folder named “SignalR” within my project and I'm heading to add a brand new category there named “EventMessageHandler”.
public class EventMessageHandler : IHandleMessages<MyMessage>
{
public void Handle(MyMessage message)
{
}
}
When the NServiceBus has revealed an event of kind “MyMessage”, “Handle” method in “EventMessageHandler” class can tackle it. For the moment, lets maintain the “Handle” method empty and let’s add a OWIN Startup class towards the project by right clicking upon the App_Start folder and selecting a OWIN Startup class template.
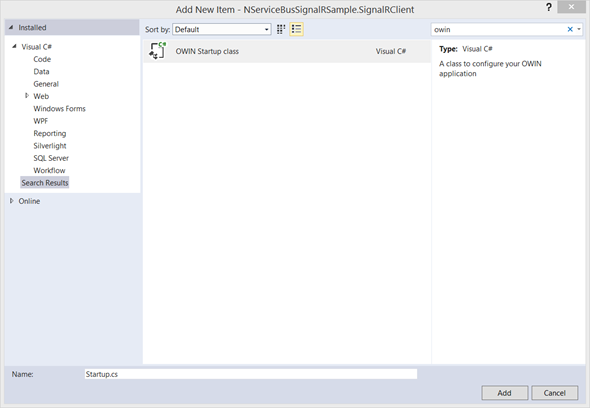
Next step, I am modifying the created OWIN Startup class with these code:
using Owin;
using Microsoft.Owin;
using NServiceBus;
[assembly: OwinStartup(typeof(NServiceBusSignalRSample.Startup))]
namespace NServiceBusSignalRSample
{
public class Startup
{
public static IBus Bus { get; private set; }
public static void Configuration(IAppBuilder app)
{
Bus = Configure
.With()
.DefaultBuilder()
.DefiningEventsAs(t => t.Namespace != null && t.Namespace.StartsWith("NServiceBusSignalRSample.Messages"))
.PurgeOnStartup(false)
.UnicastBus()
.LoadMessageHandlers()
.CreateBus()
.Start();
app.MapSignalR();
}
}
}
Right listed below I've configured my NServiceBus host and I'm mapping SignalR hubs towards the app builder pipeline. Next action is to make a SignalR hub. For the I'm adding the listed class towards the previously produced “SignalR” folder.
public class MyHub : Hub{
public void Send(string name, string message)
{
Clients.All.addNewMessageToPage(name, message);
}
}
Currently right listed below I'm deriving “MyHub” class from “Hub” and I ve got a method there named “Send” that takes 2 parameters. Within the method, I'm calling the consumer aspect “addNewMessageToPage” that still I haven’t wrote.
Just before starting off using the client aspect code, let’s modify the “Handle” method in “EventMessageHandler” class now to call my hubs’ send method.
public class EventMessageHandler : IHandleMessages<MyMessage>
{
public void Handle(MyMessage message)
{
IHubContext hubContext = GlobalHost.ConnectionManager.GetHubContext<MyHub>();
hubContext.Clients.All.addNewMessageToPage(message.Message, "Peter");
}
}
Next, I'm ready along with the back end code. Final thing to become done is to make a view in client side, initiate the hub connection and build a method named “addNewMessageToPage”. For the first I'm modifying the “HomeController” and adding a brand new Action there named “SignalR”.
public ActionResult SignalR()
{
return View();
}
Then I'm correct clicking upon the Motion and making a new view along with a similar name “SignalR”. I'm modifying the “SignalR” view as follows.
@{
ViewBag.Title = "SignalR";
}
<h2>SignalR</h2>
<div class="container">
<ul id="output"></ul>
</div>
@section scripts {
<script src="~/Scripts/jquery.signalR-2.1.1.min.js"></script>
<script src="~/signalr/hubs"></script>
<script>
$(function () {
$.connection.hub.logging = true;
$.connection.hub.start();
var chat = $.connection.myHub;
chat.client.addNewMessageToPage = function (message, name) {
$('#output').append('<li>' + message + ' ' + name + '</li>');
};
});
</script>
}
Now I'm ready. One last factor to become done is to line multiple startup projects.
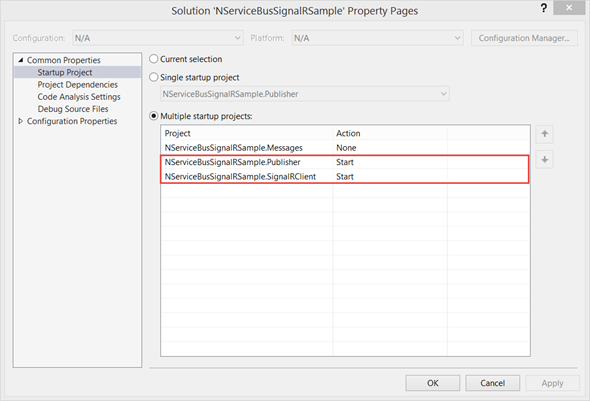
Finally, I'm running the Apps. Hope this post works for you!