
August 26, 2020 09:28 by
Peter
This article explains how to call a web API from another project using C# instead of making an Ajax call. I'm creating a web API in MVC in project1 and want to call this API in another project (like.MVC,Asp.net,.core etc) project but don't want to make any Ajax requests.
So let's see how to make a C# request for an Api Call.
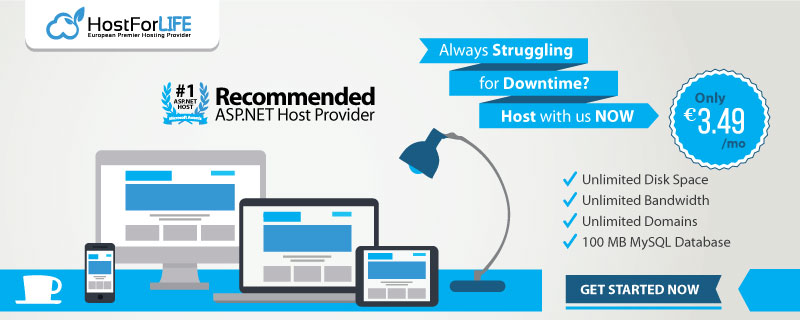
Here I am creating an API in MVC for getting a statelist in Project 1.
public class StateController : ApiController
{
[HttpGet]
[Route("api/State/StateList")]
public List<StateDto> StateList()
{
List<StateDto> StateList = new List<StateDto>();
SqlConnection sqlConnection = new SqlConnection();
string connectionString = ConfigurationManager.ConnectionStrings["Connection"].ConnectionString;
SqlCommand sqlCommand = new SqlCommand();
sqlConnection.ConnectionString = connectionString;
sqlCommand.CommandType = CommandType.Text;
sqlCommand.CommandText = "Select * From lststate where deletedbyid is null";
sqlCommand.Connection = sqlConnection;
sqlConnection.Open();
DataTable dataTable = new DataTable();
dataTable.Load(sqlCommand.ExecuteReader());
sqlConnection.Close();
if (dataTable != null)
{
foreach (DataRow row in dataTable.Rows)
{
StateList.Add(new StateDto
{
Id = (int)row["id"],
StateCode = row["Statecode"].ToString(),
StateName = row["StateName"].ToString(),
CompanyId = (int)row["Companyid"],
CreatedDate = (DateTime)row["CreatedDate"]
});
}
return StateList;
}
else
{
}
}
Project 2 where we want to call this API.
public List<StateDto> StateIndex()
{
var responseString = ApiCall.GetApi("http://localhost:58087/api/State/StateList");
var rootobject = new JavaScriptSerializer().Deserialize<List<StateDto>>(responseString);
return rootobject;
}
ApiCall.cs class
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using System.Threading.Tasks;
namespace MaheApi.Dto
{
public static class ApiCall
{
public static string GetApi(string ApiUrl)
{
var responseString = "";
var request = (HttpWebRequest)WebRequest.Create(ApiUrl);
request.Method = "GET";
request.ContentType = "application/json";
using (var response1 = request.GetResponse())
{
using (var reader = new StreamReader(response1.GetResponseStream()))
{
responseString = reader.ReadToEnd();
}
}
return responseString;
}
public static string PostApi(string ApiUrl, string postData = "")
{
var request = (HttpWebRequest)WebRequest.Create(ApiUrl);
var data = Encoding.ASCII.GetBytes(postData);
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = data.Length;
using (var stream = request.GetRequestStream())
{
stream.Write(data, 0, data.Length);
}
var response = (HttpWebResponse)request.GetResponse();
var responseString = new StreamReader(response.GetResponseStream()).ReadToEnd();
return responseString;
}
}
}
Here we get data in StateIndex method
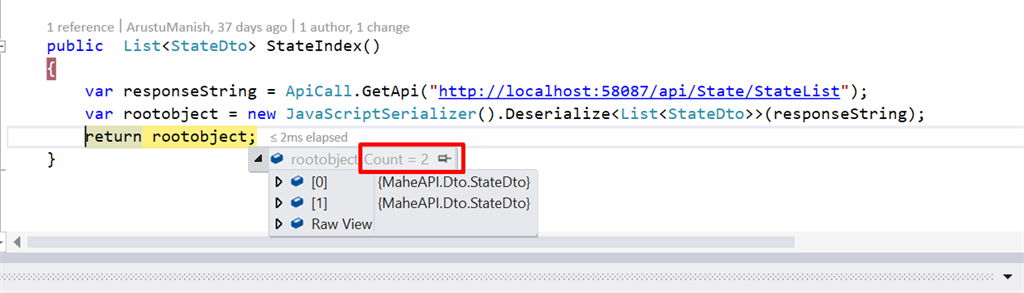