
July 23, 2024 08:21 by
Peter
Providing prompt and efficient communication is essential in the quickly changing digital world of today, particularly for educational platforms and technical blogs that serve programmers, students, and IT professionals. Similar to C# Corner, Codingvila is a technical blog that has made a name for itself as a place where computer enthusiasts can go to get help through tutorials, articles, and community assistance. The implementation of an AI chatbot can transform the way users engage with the platform and improve this support system. In order to provide real-time support and interactive learning opportunities, the "Codingvila Chatbot" makes use of.NET Core.
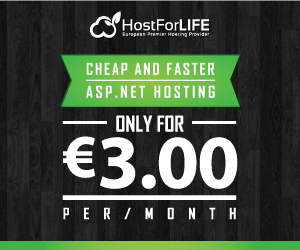
Why a Chatbot?
- Instant Support: A chatbot can provide immediate answers to common queries, reducing wait times and improving user satisfaction.
- Scalability: As the user base grows, a chatbot can effortlessly handle multiple queries at once, unlike human counterparts.
- 24/7 Availability: It offers round-the-clock support, crucial for users in different time zones or those working on projects outside typical office hours.
- Personalized Learning: The chatbot can recommend articles and tutorials based on the user's past interactions and preferences.
- Community Engagement: By handling routine questions, the chatbot allows community managers to focus on more complex queries and community-building activities.
Setting Up the Project
Ensure you have the .NET Core SDK installed to begin. Set up a new project using the command line.
dotnet new console -n HostforlifeChatbot
cd HostforlifeChatbot
This creates a basic .NET Core console application which serves as the foundation for our chatbot.
Integrating Microsoft Bot Framework
Utilize Microsoft's Bot Framework for robust chatbot functionalities. Install the necessary packages.
dotnet add package Microsoft.Bot.Builder
dotnet add package Microsoft.Bot.Builder.Integration.AspNet.Core
These tools enable the use of advanced features like dialogues and conversation flows, essential for an interactive chatbot.
Creating the Bot
1. Bot Framework Setup
Implement the HostforlifeBot.cs to manage interactions.
using Microsoft.Bot.Builder;
using Microsoft.Bot.Schema;
using System.Threading.Tasks;
public class HostforlifeBot : IBot
{
public async Task OnTurnAsync(ITurnContext turnContext, CancellationToken cancellationToken = default)
{
if (turnContext.Activity.Type == ActivityTypes.Message)
{
string userInput = turnContext.Activity.Text;
string response = ProcessInput(userInput);
await turnContext.SendActivityAsync(MessageFactory.Text(response), cancellationToken);
}
}
private string ProcessInput(string input)
{
if (input.Contains("hello"))
{
return "Hello! Welcome to Hostforlife.com, How can I assist you today?";
}
else if (input.Contains("help"))
{
return "Here are some things you can ask me...";
}
else
{
return "I'm not sure how to help with that, but I'm learning more every day!";
}
}
}
2. Configure Services and Middleware
Adjust Startup.cs to set up services for the bot.
public void ConfigureServices(IServiceCollection services)
{
services.AddBot<HostforlifeBot>(options =>
{
options.State.Add(new ConversationState(new MemoryStorage()));
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseDefaultFiles();
app.UseStaticFiles();
app.UseBotFramework();
}
Running the Chatbot
Execute the application
dotnet run
Your chatbot, "Hostforlife Chatbot" is now operational locally. It can be extended to support various platforms like Microsoft Teams, Slack, or Facebook Messenger.
Summary
The Hostforlife Chatbot, developed with .NET Core and Microsoft Bot Framework, is designed to enhance the educational resources of Hostforlife by providing an interactive, responsive, and engaging user experience. This AI-driven tool not only supports real-time communication but also encourages an interactive learning environment, making technology more accessible and comprehensible to its audience.