The Api.http file in a .NET project is a text file containing one or more HTTP requests that can be executed directly from the development environment, such as Visual Studio or Visual Studio Code. This file enables developers to quickly test API endpoints without the need for external tools such as Postman or cURL. It is particularly useful when you are debugging, developing, or testing an API. This article will describe what Api.http is and how to use it, along with an example.
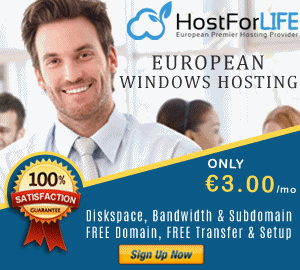
What is Api.http?
It’s a simple text file with a .http extension that contains the definitions of HTTP requests (such as GET, POST, PUT, DELETE, etc.).
Purpose: It allows developers to send HTTP requests directly from their code editor for testing API endpoints, simulating client requests, and checking API responses.
File Format: It contains HTTP requests along with headers and request bodies. This is similar to how requests are made using tools like Postman or cURL.
Benefits of using Api.http file
Quick API testing
You can make HTTP requests directly from your development environment without having to switch to external tools such as Postman.
This provides a faster feedback loop during API development.
Easy debugging
You can simulate client requests and test your API directly without writing a separate client or using a browser.
When working with RESTful services, you can easily test endpoints, troubleshoot problems, and validate responses.
Lightweight and simple
The .http file is lightweight and easy to set up. It doesn’t require complex configuration or external dependencies.
You can keep all your requests in one file or split them into different files based on API resources or services.
Version control
Because the .http file is just a text file, you can commit it to version control (like Git) along with your project.
This allows teams to share API requests and helps document the expected API inputs and outputs for specific endpoints.
Multiple requests in one file
You can write multiple requests in a single .http file, separating them with comments e.g. ###.
This is useful for testing different aspects of an API, for example, retrieving data, posting data, and updating data in one go.
Environment variables (in Visual Studio Code with the REST client)
In VS Code, you can use environment variables to avoid hardcoding values such as API tokens, endpoints, or other dynamic data, making it easier to move between development, staging, and production environments.
Use case example
Suppose you’re working on a .NET API that manages users. You want to test your API endpoints during development. Instead of using Postman or writing a front client, you can use the Api.http file to test different endpoints directly from your code editor.
Example flow
We can
- Add a GET request to retrieve a list of users.
- Add a POST request to create a new user with a JSON payload.
- Add a PUT request to update user information.
- Add a DELETE request to remove a user.
All of these can be executed directly from the Api.http file. This provides immediate feedback on the functionality of the API.
Structure of http file
The http file contains multiple requests with multiple api calls. Now, let’s go for the structure of the Api.http file. Below is a sample Api.http file.
### Get Users
GET https://api.example.com/users
Authorization: Bearer YOUR_TOKEN
### Create a New User
POST https://api.example.com/users
Content-Type: application/json
Authorization: Bearer YOUR_TOKEN
{
"name": "John Doe",
"email": "[email protected]"
}
### Update a User
PUT https://api.example.com/users/123
Content-Type: application/json
Authorization: Bearer YOUR_TOKEN
{
"name": "Jane Doe",
"email": "[email protected]"
}
### Delete a User
DELETE https://api.example.com/users/123
Authorization: Bearer YOUR_TOKEN
How to create Api.http file?
If you create a new WebAPI project in .NET 8 using Visual Studio 2022, select the WebAPI default project template, then it will automatically create YourPeojectname.http file where you can add your API requests.
However, we can add it manually for existing projects as well. To add the http file manually, follow the below steps:
Step 1. Adding http file
For Visual Studio – Right-click on your project in Solution Explorer.
Select Add > New Item and choose a Text File or File. Rename it to Api.http.
For Visual Studio code– Right-click on your project folder, select New File and name it Api.http.
Step 2. Write Http Request
Now, we can add HTTP requests to the Api.http file. Each request can be a GET, POST, PUT, or DELETE with optional headers and body, like in the example above.
Step 3. Running the request
In Visual Studio 2022
Open the Api.http file.
Visual Studio automatically detects the HTTP requests in the file. For each request, you will see a Send Request button below.

Then click on the Send Request button to execute the request.
The response status code, headers, and body will be displayed in the output pane.
In Visual Studio Code
Install the REST Client extension.
Open the Api.http file.
Hover over the HTTP request you want to execute and click on the Send Request button.
The response will be shown in a separate tab or panel.
Step 4. Check the Response
When you send the request, you can view the response in your editor, which includes:
HTTP status code e.g., 200, 404, 500.
Response body JSON, XML, or other formats.
Headers such as Content-Type, Authorization, etc..
Below is an example output of the Get Weather Forecast API.

Conclusion
The Api.http file is a powerful tool that allows you to test and debug API endpoints directly within your development environment. It provides a lightweight and integrated alternative to external tools such as Postman and can be a significant time saver when it comes to API development and testing in .NET projects.