
November 3, 2014 10:38 by
Peter
In this tutorial, I’ll explain How to Display Local Current Time using JavaScript in ASP.NET 4.5.2 without page refresh in your website. We can show a clock which is able to be showing the local time of the client’s laptop by using JavaScript.
Show/Display local computer Time use JavaScript
Following is the JavaScript function to indicate current time on webpage like clock:
<script type="text/javascript">
function DisplayCurrentTime() {
var dt = new Date();
var refresh = 1000; //Refresh rate 1000 milli sec means 1 sec
var cDate = (dt.getMonth() + 1) + "/" + dt.getDate() + "/" + dt.getFullYear();
document.getElementById('cTime').innerHTML = cDate + " – " + dt.toLocaleTimeString();
window.setTimeout('DisplayCurrentTime()', refresh);
}
</script>
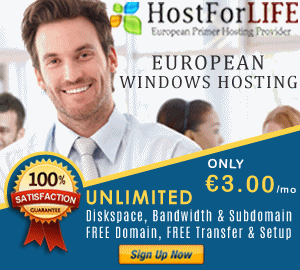
Here is the complete code for your .aspx site:
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Webblogsforyou.com | Show/Display Local Computer's Current time in webpage using
JavaScript </title>
<script type="text/javascript">
function DisplayCurrentTime() {
var dt = new Date();
var refresh = 1000; //Refresh rate 1000 milli sec means 1 sec
var cDate = (dt.getMonth() + 1) + "/" + dt.getDate() + "/" + dt.getFullYear();
document.getElementById('cTime').innerHTML = cDate + " – " + dt.toLocaleTimeString();
window.setTimeout('DisplayCurrentTime()', refresh);
}
</script>
</head>
<body onload="DisplayCurrentTime();">
<form id="form1" runat="server">
<h4>
Show/Display Local Computer's Current time in webpage using JavaScript</h4>
<div>
<asp:Label ID="cTime" runat="server" ClientIDMode="Static" BackColor="#ffff00"
Font-Bold="true" />
</div>
</form>
</body>
</html>
As you'll be able to see from on top of sample code, I called DisplayCurrentTime() method on onload event of body tag that loads this local system time, set the interval for each one seconds and refresh current time without page refresh to show on the webpage.