The programming approach known as "code-behind" allows for a more tidy division of responsibilities by separating the HTML code and display logic. It entails putting the code in a different class file, which can make it easier to comprehend and manage.
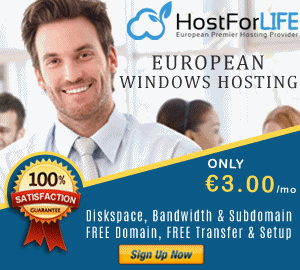
Most people associate Microsoft web forms with Code-Behind. Please be aware that the CodeBehind framework has nothing to do with Microsoft's previous web form; rather, it is a back-end framework for.NET Core. With the Code-Behind pattern, the server-side and client-side developers carry out the development work independently of each other, fully separating the design (such as HTML) from the server-side coding portion.
The issue of conditionals and loops
Please be aware that unless the server part code is added, variables such as those between the design part code are still regarded as Code-Behind.
It is quite obvious when variables are included between html or xml codes, etc.
Variable examples between the design section
Razor syntax
<h1>@model.PageName</h1>
Standard syntax
<h1><%=model.PageName%></h1>
The @model in the previous two cases.The Code-Behind method is still in place because PageName and <%=model.PageName%> data are clearly visible in the html tags and are easily recognizable by designers or client-side developers.
This is built on the Code-Behind design, thus there won't be any issues.
However, in most cases, you should either add the server side codes in the design part or the server side codes in the design part for recurring sections like loops and conditions.
Therefore, there is no way out!
In the design part is an illustration of server-side coding.
<table>
<thead>
<tr>
<th>Column1</th>
<th>Column2</th>
<th>Column3</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>@item.Column1</td>
<td>@item.Column2</td>
<td>@item.Column3</td>
</tr>
}
</tbody>
</table>
Example of integration of the design part in the server-side codes
model.ListValue = "<ul>";
foreach(string s in List)
model.ListValue += "<li>" + s + "</li>";
model.ListValue += "</ul>";
Using Code-Behind Compliance Templates
Microsoft's web form was one of the most well-known frameworks that ensured the separation of server-side scripts from the design department. However, this guarantee came at a high cost, with extra codes and limited designer control over the design department.
The most recent version of the CodeBehind framework includes a new recursion template feature that allows for the development of a 100% Code-Behind based design structure.
A page view's templates are segments that are linked to template variables prior to the compilation of the view section.
Both standard and razor syntax use the template. It is not possible to utilize the standard syntax template for razor syntax or the razor syntax template for standard syntax.
A basic template example using standard syntax.
<%@ Page Controller="YourProjectName.DefaultController" Model="YourProjectName.DefaultModel" %>
<!DOCTYPE html>
<html>
<head>
+ <#GlobalTags#>
<title><%=model.PageTitle%></title>
</head>
<body>
<%=model.BodyValue%>
</body>
</html>
+<#GlobalTags
+<meta charset="utf-8" />
+<meta name="viewport" content="width=device-width, initial-scale=1.0" />
+<meta http-equiv="Content-Type" content="text/html; charset=utf-16" />
+<meta http-equiv="content-language" content="en">
+<script type="text/javascript" src="/client/script/global.js" ></script>
+<link rel="alternate" type="application/rss+xml" title="rss feed" href="/rss/" />
+<link rel="shortcut icon" href="/favicon.ico" />
+<link rel="stylesheet" type="text/css" href="/client/style/global.css" />
+#>
The output is translated as follows in this example when the Template variable calls a Template section.
<%@ Page Controller="YourProjectName.DefaultController" Model="YourProjectName.DefaultModel" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-16" />
<meta http-equiv="content-language" content="en">
<script type="text/javascript" src="/client/script/global.js" ></script>
<link rel="alternate" type="application/rss+xml" title="rss feed" href="/rss/" />
<link rel="shortcut icon" href="/favicon.ico" />
<link rel="stylesheet" type="text/css" href="/client/style/global.css" />
<title><%=model.PageTitle%></title>
</head>
<body>
<%=model.BodyValue%>
</body>
</html>
The aforementioned example introduced you to the idea of templates and demonstrated their basic functionality in the CodeBehind framework. However, return templates have one special feature: they are first put into the template before replacing the entire template.
Two categories of templates exist.
Template: Return to template
The template variable can be used to call either type of template.
A template definition example using Razor syntax.
+@#TemplateName{
@{
string ExampleText = "This is example text";
}
<b>Example value is : @ExampleText</b>
+}
In each template definition, the at sign and sharp (@#) characters are used first, followed by the template name, and finally the block character ({) at the conclusion. The block is closed (}) when the desired values have been added.The template variable must be written in order to call the template. The name of the template is typed after the sign and sharp (@#) letters are added at the beginning to write the template variable.Example of using a template variable to call a template.
<!DOCTYPE html>
<html>
<body>
+ @#TemplateName
</body>
</html>
Example of after pasting the template.
<!DOCTYPE html>
<html>
<body>
@{
string ExampleText = "This is example text";
}
<b>Example value is : @ExampleText</b>
</body>
</html>
Returned templates are first inserted into a variable with the same name as the template name, and then the entire template is replaced by the returned template (all three are the same name).
The at sign and sharp (@#) characters are used at the beginning of each return template definition, and then the name of the template is written. Then, the equal character (=) is added, and at the end, the block character ({) is used. Then, the desired values are added, and finally, the block is closed (}).
Example (Razor syntax)View the section before pasting the template.
In the codes above, there is a return template called Tags, which is specified with the string @#Tags= and its value is known inside the blockpPasting template auto step 1.
<div class="header">
<a href="#default">@model.Title</a>
<div class="header-right">
@#Tags={<a href="@#Href">@#PageName</a>}
</div>
</div>
@#Tags{
@foreach (PageItem page in model.PageItemList)
{
<a href="@#Href">@#PageName</a>
}
}
@#PageName{@page.Title}
@#Href{@(((page.Path == "main")? "/" : page.Path))}
As you can see in the above codes. First, the value inside the returned template block with the name Tags is replaced in the Tags template variable inside the Tags template.
pasting template auto step 2.
<div class="header">
<a href="#default">@model.Title</a>
<div class="header-right">
@foreach (PageItem page in model.PageItemList)
{
<a href="@#Href">@#PageName</a>
}
</div>
</div>
@#PageName{@page.Title}
@#Href{@(((page.Path == "main")? "/" : page.Path))}
According to the above codes, in the second step, the values of the Tags template are replaced in the place of the returned template.
pasting template auto step 3 (finally).
<div class="header">
<a href="#default">@model.Title</a>
<div class="header-right">
@foreach (PageItem page in model.PageItemList)
{
<a href="@(((page.Path == "main")? "/" : page.Path))">@page.Title</a>
}
</div>
</div>
In the last step, the values of other templates are replaced in the place of template variables.
In the template placement example, if you add the template externally, you will have the following html in the design section.
View the section before pasting the template.
<div class="header">
<a href="#default">@model.Title</a>
<div class="header-right">
+ @#Tags={<a href="@#Href">@#PageName</a>}
</div>
</div>
Without a return template, at best, you will have the following html in the design section.
View the section without the use of the return template.
<div class="header">
<a href="#default">@model.Title</a>
<div class="header-right">
+ @foreach (PageItem page in model.PageItemList)
+ {
+ <a href="@(((page.Path == "main")? "/" : page.Path))">@page.Title</a>
+ }
</div>
</div>
Please note that this was a simple example, and the situation may be very complex.
You can also use a template for standard syntax. The following code shows the previous template example in standard syntax.
<div class="header">
<a href="#default"><%=model.Title%></a>
<div class="header-right">
<#Tags=<a href="<#Href#>"><#PageName#></a>#>
</div>
</div>
<#Tags
<% foreach (PageItem page in model.PageItemList)
{
<#Tags#>
}
%>
#>
<#PageName <%=page.Title%>#>
<#Href <%=((page.Path == "main")? "/" : page.Path)%>#>
If the return value is placed between the standard syntax tags, the syntax will be automatically closed before the return value, and the syntax will be opened after it.
Copy template auto step finally for standard syntax.
<div class="header">
<a href="#default"><%=model.Title%></a>
<div class="header-right">
<% foreach (PageItem page in model.PageItemList)
{
%><a href="<%=((page.Path == "main")? "/" : page.Path)%>"><%=page.Title%></a><%
}
%>
</div>
</div>
Conclusion
No matter how much you try to avoid mixing server-side codes in the view section, in some cases, such as loops and conditions, you will have to use server-side codes in the view section; the presence of mixing server-side codes reduces the readability of the codes and makes the appearance of the view section ugly, and it becomes difficult to maintain the codes and also leads to complex interaction between the server-side and client-side developers in high-scale applications. Applying the return template keeps the code of the server section outside the view section. As a result, the view section will have a great appearance, and the Code-Behind pattern will be fully respected.