
October 20, 2014 06:29 by
Peter
ASP.NET 4.5.2’s file upload facility offers a quick, easy method for allowing users to upload content to your server. In many cases however the upload type must be restricted. For example, perhaps you are allowing users to upload a profile image. You certainly don’t want them to be capable of uploading an executable (.exe) file.
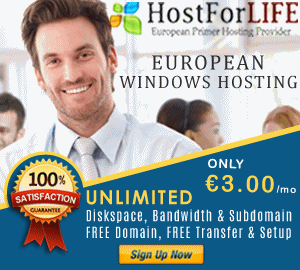
The simple solution is to attach a RegularExpressionValidator to the file upload control. Below is an example of a RegularExpressionValidator restricting the accepted filetypes of a FileUpload control to .gif, .jpg, .jpeg or .png
<asp:FileUpload ID="uplImage" runat="server" />
<asp:RegularExpressionValidator ID="revImage" ControlToValidate="uplImage" ValidationExpression="^.*\.((j|J)(p|P)(e|E)?(g|G)|(g|G)(i|I)(f|F)|(p|P)(n|N)(g|G))$" Text=" ! Invalid image type" runat="server" />
Here is the regular expression.
^.*\.((j|J)(p|P)(e|E)?(g|G)|(g|G)(i|I)(f|F)|(p|P)(n|N)(g|G))$
Unfortunately we cannot merely ignore case in the expression. The RegularExpressionValidator doesn't have an option to ignore case and the ASP.NET Regex Engine’s ignore case construct isn't supported on the client side Javascript Regex Engine. However, the expression on top of can match all variations of upper/lowercase characters like .gif, .Gif and .GIF
Don’t forget to see in your postback handler that the page is valid! If you are doing forget and therefore the regular expression match fails, your code can still execute.
protected void btnUpload_Click(object sender, EventArgs e)
{
if (IsValid)
{
// Process your data
}
}