Because of its simplicity, statelessness, and versatility, JWT (JSON Web Token) authentication has become a popular way for securing APIs and web applications. In this post, we'll look at how to use JWT authentication with ASP.NET Core, a powerful framework for creating modern web apps.
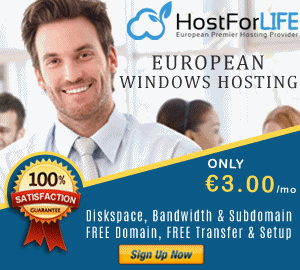
JWT Authentication Explained
JWT is a concise and self-contained method of transmitting JSON-formatted information between parties. It is made up of three sections: a header, a payload, and a signature. Typically, the header contains information about the token, such as its type and the hashing algorithm employed. The payload holds the claims or data linked with the token, whereas the signature is used to validate the token's integrity.
The Advantages of JWT Authentication
- JWT tokens are stateless since they are self-contained and do not require the server to keep session information. As a result, JWT authentication is well suited to scalability and microservices designs.
- Decentralized: Because the token contains all of the required information, the authentication procedure is not reliant on centralized authentication servers.
- JWT tokens are secure since the signature verifies that the information has not been tampered with.
ASP.NET Core JWT Authentication Implementation
Step 1: Begin by creating a new ASP.NET Core project. Begin by launching a new ASP.NET Core project using the specified template.
Step 2: Install the Necessary Packages Install the following packages with NuGet Package Manager: System and Microsoft.AspNetCore.Authentication.JwtBearer.IdentityModel.Tokens.Jwt.
Step 3: Set up Authentication To configure JWT authentication, add the following code to the Startup.cs file inside the ConfigureServices method:
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
})
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = "your-issuer",
ValidAudience = "your-audience",
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your-secret-key"))
};
});
Step 4: Secure Your API Endpoints Add the [Authorize] attribute to the necessary controllers or actions to protect your API endpoints using JWT authentication.
Step 5: Create JWT Tokens You must produce a JWT token and return it to the client when a user logs in. To create and sign tokens, you can use libraries such as System.IdentityModel.Tokens.Jwt.
HostForLIFE.eu ASP.NET 8 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
