
July 17, 2024 08:49 by
Peter
Web application security relies heavily on authentication and authorization to make sure users are who they say they are and to control what actions they can do. Robust means for integrating authorization and authentication into your apps are provided by ASP.NET Core. We'll go into great detail about these ideas in this post, including key elements, recommended procedures, and real-world application examples.
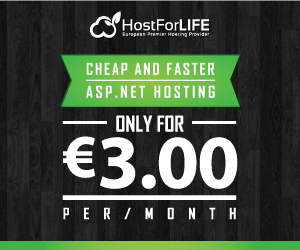
Understanding Authentication and Authorization
Authentication verifies the identity of users attempting to access your application. It answers the question, "Who are you?" Common authentication methods include.
- Cookie-based Authentication: Uses encrypted cookies to authenticate users.
- Token-based Authentication: Utilizes JWT (JSON Web Tokens) or OAuth tokens for authentication.
- External Authentication Providers: Allows users to log in using external providers like Google, Facebook, etc.
- Windows Authentication: Uses Windows credentials for intranet applications.
Authorization, on the other hand, determines what authenticated users are allowed to do within the application. It answers the question, "What are you allowed to do?" Authorization can be role-based, policy-based, or claim-based, where.
Role-based Authorization: Assigns roles (admin, user, manager) to users and restricts access based on roles.
Policy-based Authorization: Defines access policies that evaluate a combination of roles, claims, and requirements.
Claim-based Authorization: Grants access based on specific claims associated with the user's identity.
Implementing Authentication in ASP.NET Core
Cookie Authentication: Cookie authentication is commonly used for web applications that require user sessions. ASP.NET Core provides built-in middleware to handle cookie-based authentication.
// Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme)
.AddCookie(options =>
{
options.Cookie.Name = "YourAppCookie";
options.LoginPath = "/Account/Login";
options.AccessDeniedPath = "/Account/AccessDenied";
});
}
Token-based Authentication: Token-based authentication is suitable for APIs and single-page applications (SPA). ASP.NET Core supports JWT (JSON Web Tokens) authentication out of the box.
// Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = Configuration["Jwt:Issuer"],
ValidAudience = Configuration["Jwt:Issuer"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:Key"]))
};
});
}
Implementing Authorization in ASP.NET Core: Authorization is configured using policies and requirements. Define policies in Startup.cs and apply them using the [Authorize] attribute in controllers or actions.
// Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthorization(options =>
{
options.AddPolicy("AdminOnly", policy => policy.RequireRole("Admin"));
options.AddPolicy("MinimumAge", policy =>
policy.Requirements.Add(new MinimumAgeRequirement(18)));
});
}
// Controller
[Authorize(Policy = "AdminOnly")]
public IActionResult AdminDashboard()
{
return View();
}
Summary
In this article, we've explored the fundamentals of authentication and authorization in ASP.NET Core. Understanding these concepts is crucial for building secure and scalable web applications. ASP.NET Core provides flexible and powerful tools to implement various authentication and authorization mechanisms tailored to your application's needs. By leveraging these capabilities effectively, you can ensure that your application remains secure and compliant with modern security standards.
Implementing authentication and authorization involves configuring middleware, defining policies, and applying attributes correctly across your application. Whether you choose cookie-based authentication for web applications or token-based authentication for APIs, ASP.NET Core offers comprehensive support and flexibility to meet your security requirements.
By following best practices and staying updated with the latest security trends, you can build robust and secure ASP.NET Core applications that protect user data and maintain user trust.