A cloud-ready, opinionated stack for developing distributed, observable, production-ready applications is.NET Aspire. Delivery of.NET Aspire occurs via a group of NuGet packages that address particular cloud-native issues. Microservices, which are tiny, interconnected units of code, are frequently used in place of a single, large code base in cloud-native applications. Typically, cloud-native applications use a lot of resources, including messaging, databases, and caching.
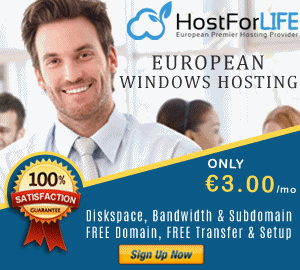
Overview
A cloud-ready toolset for creating contemporary distributed applications is called.NET Aspire. Its main goal is to assist developers in building observable, scalable microservices. .NET Aspire makes it easier to deploy.NET Core APIs and enables you to rapidly create cloud-native applications with a set of NuGet packages. Rather to having a single, massive code base, these applications usually comprise of smaller, connected components. For their operations to be successful in a cloud setting, they frequently depend on services like messaging, databases, and caching.
Starting a New Visual Studio 2022 Project
Starting a New Visual Studio 2022 Project An overview Launch the Visual Studio application, then choose "Create a new project."
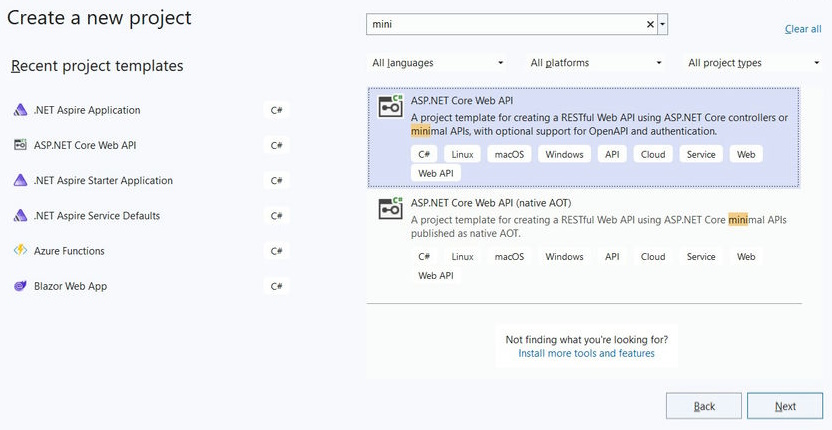
The chosen application's project structure will be created by Visual Studio 2022. Since we're utilizing the ASP.Net Core Web API in this example, we can either utilize the pre-existing controller or construct a new one to write the code. You can enter the code there and use it to create or run the program.
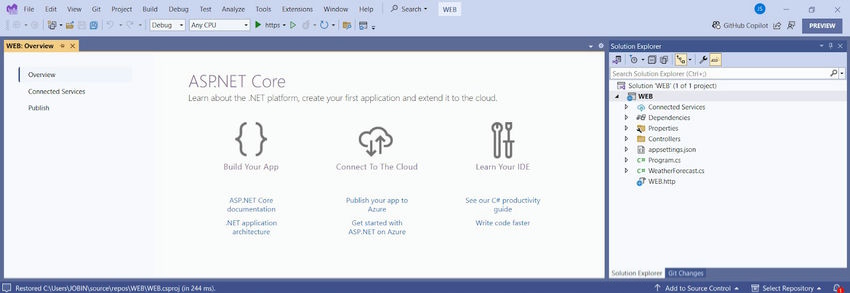
To create a .NET Aspire Application, follow these steps.
- Right-click on the Solution section in Visual Studio.
- Select "Add" > "New Project."
- Choose "Create a new project."
- Find and select ".NET Aspire Application" from the list of project templates.
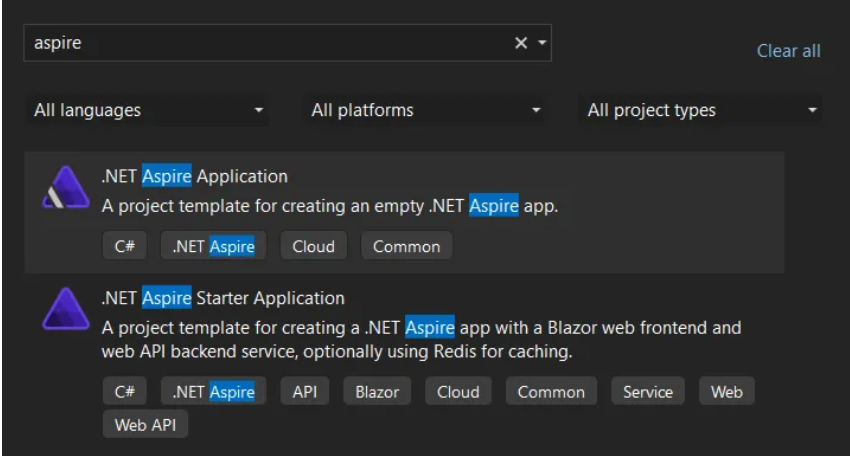
The .NET Aspire Application is a basic starter template that includes only the AppHost and ServiceDefaults projects. This template is designed to only provide the essentials for you to build off of
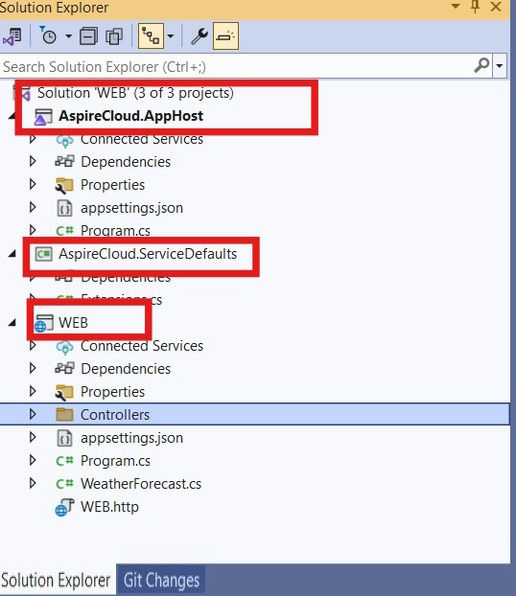
The .NET Aspire Application is a basic starter template that includes only the AppHost and ServiceDefaults projects. This template is designed to provide the essentials to build upon.
Project Structure
- WEB: This is an ASP.NET Core API project, This project depends on the shared AspireCloud.ServiceDefaults project.
- AspireCloud.AppHost: This is an orchestrator project designed to connect and configure the different projects and services of your app. This project handles running all of the projects that are part of the .NET Aspire application. The orchestrator should be set as the Startup project, and it depends on the WEBThis is the project responsible for running the applications inside of this solution.
- AspireCloud.ServiceDefaults: This is a.NET Aspire shared project to manage configurations that are reused across the projects in your solution related to resilience, service discovery, and telemetry. This project ensures that all dependent services share the same resilience, service discovery, and OpenTelemetry configuration.
In the Program.cs file on the AppHost project, you can see the following code.
var builder = DistributedApplication.CreateBuilder(args);
var RESTAPI = builder.AddProject<Projects.WEB>("RESTAPI");
builder.Build().Run();
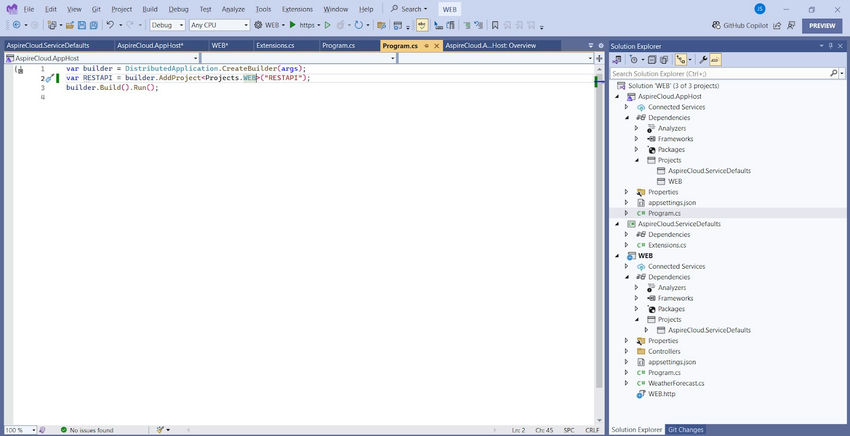
WEB .NET core API program.cs file configured in Aspire.ServiceDefaults.
builder.AddServiceDefaults();
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
//cloud configuration:
builder.AddServiceDefaults();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
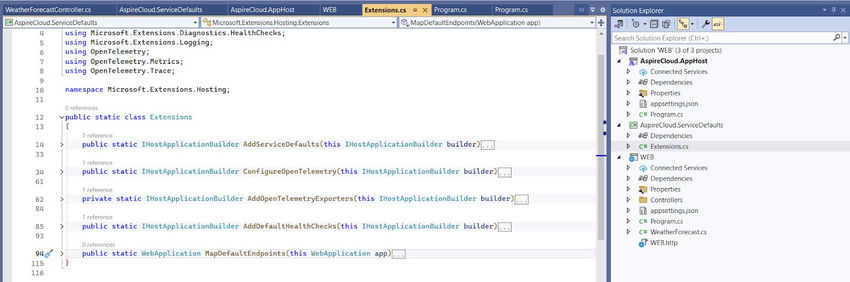
On the Extensions. cs file, you can find some extension methods such as:
- AddServiceDefaults: used to add default functionality.
- ConfigureOpenTelemetry: used to configure OpenTelemetry metrics and tracing
- AddDefaultHealthChecks: adds default health checks endpoints
- MapDefaultEndpoints: maps the health check endpoint to /health and the liveness endpoint to alive.
Dashboard
Start the project with AspireCloud.AppHost is the Starter project (this project knows how to run the whole distributed application), and the following page will be opened in your browser with this nice dashboard.
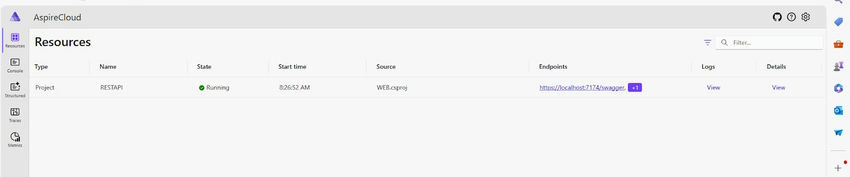
In this dashboard, you can monitor various parts of your app, such as,
- Projects: Displays information about your .NET projects in your .NET Aspire app, such as the app state, endpoints, and environment variables.
- Containers: Displays information about your app containers, such as state, image tag, and port number (you should also see the Redis container you added for output caching with the name you provided.
- Executables: Displays the running executables used by your app.
- Logs: In this section, you can see the output logs for the projects, containers, and executables, and can also see the logs in a table format (structured).
- Traces: displays the traces for your application, providing information about the requests.
- Metrics: Displays various instruments and meters that are exposed and their corresponding dimensions for your app.
The Traces for your APIs.
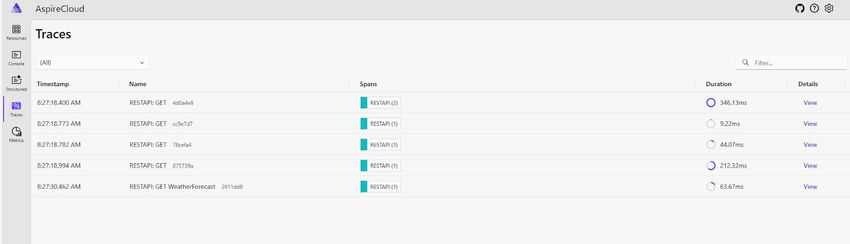